Arrays are a fundamental data structure in many programming languages, including Java. They allow for sequential storage and easy manipulation of elements, making them a popular choice for storing data. However, removing an element from an array can be a tricky task, as all elements are stored sequentially in a single memory block. In this article, we will explore some common techniques for removing elements from Java arrays, along with their pros and cons.
A Short Briefing on Arrays
Before diving into the different methods for removing elements from arrays, let’s first understand what arrays are and how they work. An array is a data structure that stores a collection of elements of the same type in a contiguous block of memory. This means that all elements are stored one after another, making it easy to access them using their index.
For example, consider an array of integers with 5 elements: [10, 20, 30, 40, 50]. Each element takes up the same amount of memory, so they are stored sequentially in memory as follows:
Index | Memory Address | Value |
---|---|---|
0 | 1000 | 10 |
1 | 1004 | 20 |
2 | 1008 | 30 |
3 | 1012 | 40 |
4 | 1016 | 50 |
When we want to access an element at a specific index, say index 2, the underlying mechanism uses pointer arithmetic to calculate the memory address of that element. In this case, it would be 1008 (memory address of the first element) + 2 * 4 (size of each integer element). This computation leads directly to the element with index 2, which is 30. This process is known as random access and has a time complexity of O(1), which means it is as fast as it can be.
Now that we have a basic understanding of how arrays work, let’s explore some techniques for removing elements from them.
Using Two Arrays
One simple approach to remove an element from an array is to create a new array with one less element and copy all the elements except the one we want to remove into it. This method is straightforward and easy to understand, but it comes at the cost of creating a new array and copying all the elements, which can be inefficient for large arrays.
Implementation
To implement this method, we first need to find the index of the element we want to remove. Once we have the index, we can create a new array with one less element and use a loop to copy all the elements from the original array into the new one, except for the element at the given index.
public static int[] removeElement(int[] arr, int index) {
// Find the length of the new array
int length = arr.length - 1;
// Create a new array with one less element
int[] newArr = new int[length];
// Copy all elements except the one at the given index
for (int i = 0, j = 0; i < arr.length; i++) {
if (i != index) {
newArr[j] = arr[i];
j++;
}
}
return newArr;
}
Example
Let’s say we have an array of integers [10, 20, 30, 40, 50] and we want to remove the element at index 2 (which is 30). Using the above method, we would get a new array with the following elements: [10, 20, 40, 50].
ArraysUtils.remove()
The ArraysUtils class in Java provides a convenient method called remove() that allows us to remove an element from an array. This method takes in the original array and the index of the element we want to remove as parameters and returns a new array with the element removed.
Implementation
To use this method, we first need to import the ArraysUtils class from the java.utilpackage. Then, we can simply call the remove() method and pass in the original array and the index of the element we want to remove.
import java.util.ArraysUtils;
public static int[] removeElement(int[] arr, int index) {
return ArraysUtils.remove(arr, index);
}
Example
Using the same example as before, if we want to remove the element at index 2 (which is 30) from the array [10, 20, 30, 40, 50], we would get a new array with the following elements: [10, 20, 40, 50].
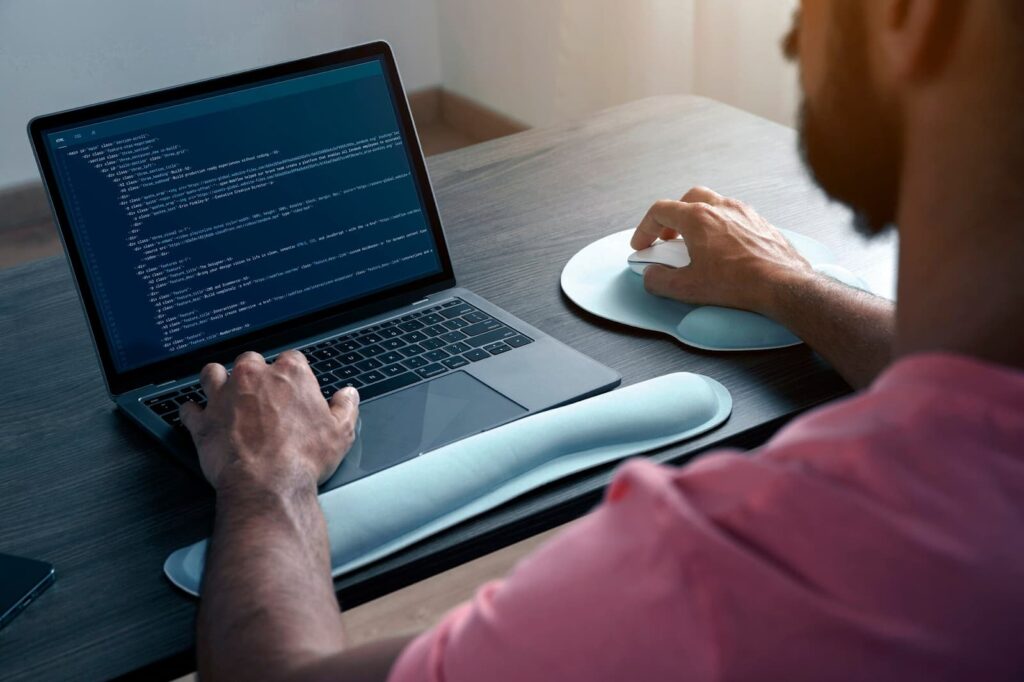
Using a for loop
Another approach to removing an element from an array is to use a for loop to iterate through the array and shift all the elements after the given index one position to the left. This method avoids creating a new array but requires more code and has a time complexity of O(n), where n is the number of elements in the array.
Implementation
To implement this method, we first need to find the index of the element we want to remove. Then, we can use a for loop to iterate through the array and shift all the elements after the given index one position to the left. Finally, we need to update the length of the array to reflect the removal of the element.
public static int[] removeElement(int[] arr, int index) {
// Shift all elements after the given index one position to the left
for (int i = index; i < arr.length - 1; i++) {
arr[i] = arr[i + 1];
}
// Update the length of the array
arr[arr.length - 1] = 0;
return arr;
}
Example
Using the same example as before, if we want to remove the element at index 2 (which is 30) from the array [10, 20, 30, 40, 50], we would get the same array with the following elements: [10, 20, 40, 50, 0]. Note that the last element has been set to 0, which indicates that it is no longer part of the array.
System.arraycopy()
The System class in Java provides a method called arraycopy() that allows us to copy elements from one array to another. This method can also be used to remove an element from an array by copying all the elements except the one we want to remove into a new array. However, like the first method we discussed, this approach also requires creating a new array and copying all the elements, making it inefficient for large arrays.
Implementation
To use this method, we first need to find the index of the element we want to remove. Then, we can use the arraycopy() method to copy all the elements from the original array into a new array, except for the element at the given index.
public static int[] removeElement(int[] arr, int index) {
// Shift all elements after the given index one position to the left
for (int i = index; i < arr.length - 1; i++) {
arr[i] = arr[i + 1];
}
// Update the length of the array
arr[arr.length - 1] = 0;
return arr;
}
Example
Using the same example as before, if we want to remove the element at index 2 (which is 30) from the array [10, 20, 30, 40, 50], we would get a new array with the following elements: [10, 20, 40, 50].
Conclusion
In this article, we explored four different techniques for removing elements from Java arrays. Each method has its own pros and cons, and the best approach will depend on the specific requirements of your program. The first two methods involve creating a new array, which can be inefficient for large arrays. On the other hand, the last two methods avoid creating a new array but require more code and have a time complexity of O(n). It’s important to understand these techniques and choose the one that best suits your needs.