Arrays are a fundamental concept in programming, and anyone with experience in languages like C or FORTRAN is familiar with their use. In simple terms, arrays are a contiguous block of memory where each location holds a specific type of data, such as integers or floating-point numbers. However, the situation in Java is slightly different, with some additional complexities. In this article, we will explore the process of initializing arrays in Java, discussing its syntax, purpose, and best practices. So let’s dive in and learn how to initialize an array in Java.
An Example Array
To understand the process of initializing arrays in Java, let’s start with a basic example. Consider the following code snippet:
int[] ia = new int[10];
From left to right, the above code can be broken down into the following components:
- int[] – This declares the type of the variable as an array (denoted by the []) of integers;
- ia – This is the name of the variable, which in this case is ia;
- = – This symbol indicates that the variable defined on the left side is set to what’s on the right side;
- new – In Java, the new keyword signifies that an object is being initialized, meaning that storage is allocated and its constructor is called;
- int[10] – This tells us that the specific object being initialized is an array of 10 integers.
It is worth noting that since Java is a strongly-typed language, the type of the variable iamust be compatible with the type of the expression on the right-hand side of the = sign.
Initializing the Example Array
Now that we have seen a simple example of initializing an array in Java, let’s put it into practice and see how it works. Save the following code in a file called Test1.java and use javac to compile it, followed by java to run it (in the terminal, of course):
import java.lang.*;
public class Test1 {
public static void main(String[] args) {
int[] ia = new int[10];
for (int i = 0; i < ia.length; i++) {
ia[i] = i;
}
System.out.println("The elements of the array are:");
for (int i = 0; i < ia.length; i++) {
System.out.print(ia[i] + " ");
}
}
}
Upon running the above code, you should see the following output:
The elements of the array are:
0 1 2 3 4 5 6 7 8 9
Let’s break down the code and understand what is happening. In the first line, we import the java.lang package, which contains the System class that provides access to the standard input, output, and error streams. Next, we define a class called Test1, which contains a mainmethod – the entry point for our program.
Inside the main method, we declare an integer array ia with a size of 10. Then, using a forloop, we assign values to each element of the array. The loop runs from 0 to the length of the array (ia.length) and assigns the value of i to each element. Finally, we print out the elements of the array using another for loop.
Why Do I Want to Initialize an Array, Anyway?
Now that we have seen how to initialize an array in Java, you might be wondering why we need to do it in the first place. Well, there are a few reasons why initializing an array is essential. Let’s explore them in detail.
Storing Multiple Values
One of the primary purposes of arrays is to store multiple values of the same type in a single variable. This allows us to access and manipulate these values easily. For example, if we want to store the grades of 10 students, we can use an array instead of creating 10 separate variables for each grade. This not only makes our code more organized but also reduces the chances of errors.
Efficient Memory Management
Arrays are stored in contiguous memory locations, which means that all the elements are stored one after another. This makes it easier for the computer to access and manipulate the data, resulting in faster execution times. Additionally, since arrays have a fixed size, the computer knows exactly how much memory to allocate for them, making memory management more efficient.
Flexibility in Data Manipulation
Arrays provide us with various methods to manipulate the data they hold. For instance, we can add or remove elements from an array, sort its contents, or search for specific values. This flexibility allows us to perform complex operations on our data with ease.
Taking the Array Further
So far, we have seen how to initialize a simple array of integers in Java. However, arrays can hold different types of data, and we can also create multidimensional arrays – arrays within arrays. Let’s take a look at some examples to understand this better.
Arrays of Different Types
In Java, arrays can hold any type of data, including primitive types like int and double, as well as objects. Consider the following code snippet:
String[] names = new String[5];
names[0] = "John";
names[1] = "Jane";
names[2] = "Bob";
names[3] = "Alice";
names[4] = "Mark";
In the above example, we have created an array of strings and assigned values to each element. Similarly, we can create arrays of other types, such as int[], double[], or even custom objects.
Multidimensional Arrays
Multidimensional arrays are arrays that contain other arrays as their elements. They are useful when dealing with data that has multiple dimensions, such as a matrix or a table. Let’s see an example of a 2D array in Java:
int[][] matrix = new int[3][3];
matrix[0][0] = 1;
matrix[0][1] = 2;
matrix[0][2] = 3;
matrix[1][0] = 4;
matrix[1][1] = 5;
matrix[1][2] = 6;
matrix[2][0] = 7;
matrix[2][1] = 8;
matrix[2][2] = 9;
In the above code, we have created a 2D array called matrix with a size of 3×3. We then assign values to each element using nested for loops. The first loop iterates over the rows, while the second loop iterates over the columns. This results in the following matrix:
Column 0 | Column 1 | Column 2 | |
---|---|---|---|
Row 0 | 1 | 2 | 3 |
Row 1 | 4 | 5 | 6 |
Row 2 | 7 | 8 | 9 |
We can also create multidimensional arrays with more than two dimensions, such as a 3D array or even higher. However, the concept remains the same – each element of the array is another array.
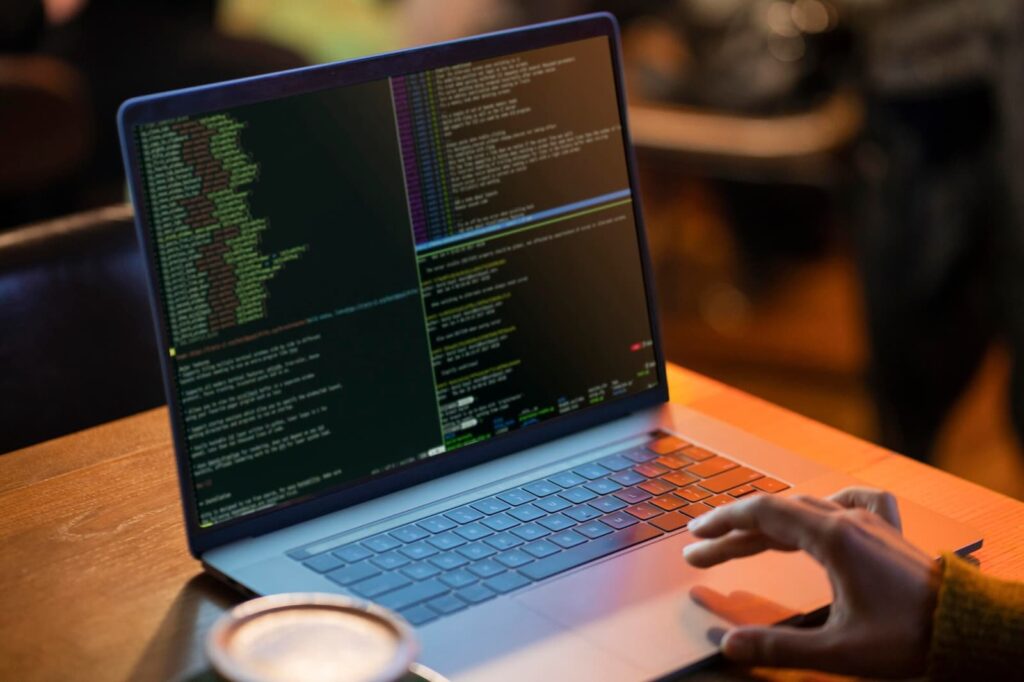
Best Practices for Initializing Arrays
Now that we have a good understanding of how to initialize arrays in Java, let’s discuss some best practices that can help us write better code and avoid common pitfalls.
Use Descriptive Variable Names
When declaring an array, it is essential to use descriptive variable names that accurately represent the data stored in the array. This not only makes our code more readable but also helps us avoid confusion when working with multiple arrays.
Initialize Arrays with Default Values
In Java, when we declare an array without explicitly assigning values to its elements, they are automatically initialized with default values. For example, an int array will have all its elements set to 0, while a String array will have all its elements set to null. It is good practice to take advantage of this feature and avoid unnecessary initialization.
Use Enhanced For Loops for Iteration
Java provides an enhanced for loop, also known as a “for-each” loop, which is useful for iterating over arrays. It simplifies the syntax and makes our code more concise. Consider the following example:
int[] numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
System.out.print(num + " ");
}
The above code is equivalent to the following traditional for loop:
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.print(numbers[i] + " ");
}
Using the enhanced for loop not only saves us from writing extra code but also reduces the chances of errors.
Avoid Hard-Coding Array Sizes
It is generally considered bad practice to hard-code the size of an array in our code. This makes it difficult to modify the size of the array in the future, and if we need to change it, we would have to go through our entire codebase and make the necessary changes. Instead, we can use a constant or a variable to represent the size of the array, making it easier to modify in the future.
Conclusion
In this article, we have explored the process of initializing arrays in Java, discussing its syntax, purpose, and best practices. We started with a simple example and then moved on to more complex concepts like multidimensional arrays. We also discussed why initializing arrays is essential and how it helps us write efficient and organized code. By following the best practices mentioned in this article, we can ensure that our code is easy to read, maintain, and modify. With this knowledge, you are now equipped to use arrays effectively in your Java programs.