Sorting a map by key is a common task in Java programming. A map is a data structure that stores key-value pairs, and it is often used to represent relationships between different objects. However, the elements in a map are not stored in any particular order, which can make it difficult to retrieve them in a specific sequence. In this article, we will explore different ways to sort a map by its keys in Java.
What are Maps in Java?
Before we dive into sorting a map by key, let’s first understand what maps are and how they work in Java. A map is an interface defined in the Java Development Kit (JDK), and it represents a collection of key-value pairs. It is part of the java.util package and is implemented by various classes such as HashMap, TreeMap, and LinkedHashMap.
A map allows you to store and retrieve values based on their corresponding keys. This makes it useful for representing relationships between different objects, where each object has a unique identifier or key. For example, you could use a map to store student names and their corresponding grades, with the student names being the keys and the grades being the values.
Types of Maps in Java
As mentioned earlier, there are several implementations of the Map interface in Java. Each implementation has its own unique characteristics and is suitable for different use cases. Let’s take a brief look at some of the commonly used map implementations in Java:
- HashMap: This is the most commonly used map implementation in Java. It uses a hash table to store the key-value pairs, which allows for fast retrieval of values based on their keys. However, the elements in a HashMap are not stored in any particular order;
- TreeMap: This implementation uses a red-black tree data structure to store the key-value pairs. Unlike HashMap, the elements in a TreeMap are sorted according to their keys. This makes it useful for scenarios where you need to retrieve the elements in a specific order.
Apart from these main implementations, there are also other specialized map implementations such as IdentityHashMap, RenderingHints, WeakHashMap, and more. Each of these implementations has its own unique characteristics and is suitable for specific use cases.
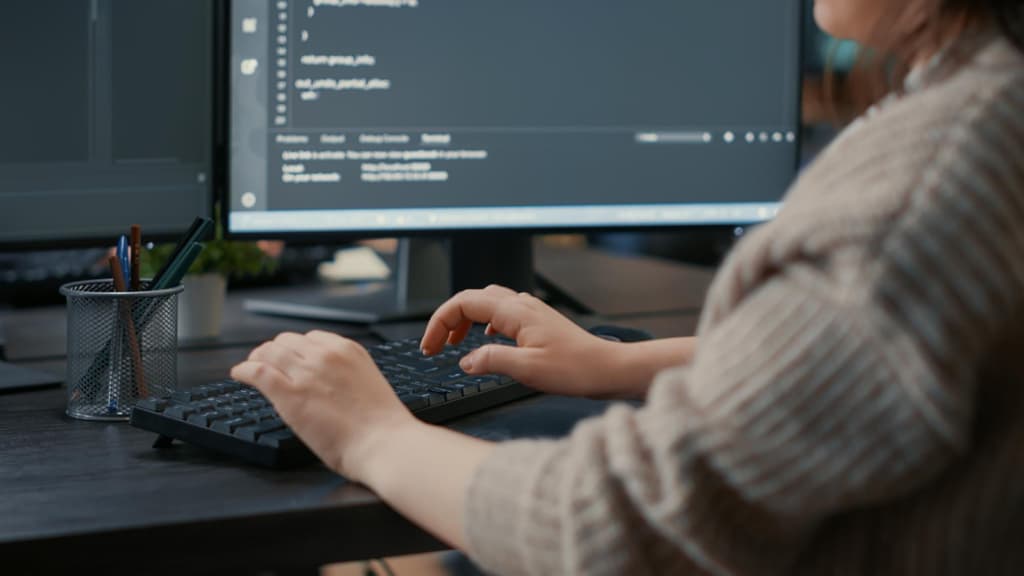
Sorting a Map by Key in Java
Now that we have a basic understanding of maps in Java, let’s explore different ways to sort a map by its keys. We will use the following map as an example throughout this article:
Map grades = new HashMap();
grades.put("John", 85);
grades.put("Mary", 92);
grades.put("Bob", 78);
grades.put("Alice", 90);
Using HashMap to Sort a Map by Key
The first approach we will look at is using the HashMap class to sort a map by key. As mentioned earlier, HashMap does not guarantee any particular order for its elements. However, we can use the keySet() method to get a set of all the keys in the map and then sort them using the Arrays.sort() method. Let’s see how this works in code:
Object[] keys = grades.keySet().toArray();
Arrays.sort(keys);
for (Object key : keys) {
System.out.println(key + ": " + grades.get(key));
}
In the above code, we first convert the set of keys into an array using the toArray() method. Then, we use the Arrays.sort() method to sort the keys in ascending order. Finally, we iterate over the sorted keys and print out the corresponding values from the map.
Using TreeMap to Sort a Map by Key
The second approach we will look at is using the TreeMap class to sort a map by key. Unlike HashMap, TreeMap maintains its elements in sorted order according to their keys. This makes it an ideal choice for sorting a map by key. Let’s see how this works in code:
TreeSet keys = new TreeSet(grades.keySet());
for (String key : keys) {
System.out.println(key + ": " + grades.get(key));
}
Conclusion
Sorting a map by key is a common task in Java programming. In this article, we explored different ways to achieve this, including using HashMap, TreeMap, and generic types. Each approach has its own advantages and is suitable for different use cases. It is important to understand the characteristics of each map implementation in order to choose the most appropriate one for your specific needs.