Entering the realm of game development requires the establishment of a conducive environment and the selection of appropriate tools. Opting for Java as the programming language means understanding its specific setups tailored for game creation.
Java Development Kit Installation: How to Make a Game in Java?
Before embarking on the development journey, installing the Java Development Kit (JDK) is paramount. This kit encompasses the essential tools, executables, and binaries needed for compiling and running Java applications.
```java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
```
Once the “HelloWorld.java” program is written, it should be saved with that name. Following this, one should navigate to the file’s directory via a terminal or command prompt, compile the file with `javac HelloWorld.java`, and execute it with `java HelloWorld`. Success is confirmed upon seeing the “Hello, World!” output, indicating a successful JDK setup.
Selecting an Integrated Development Environment
The choice of an Integrated Development Environment (IDE) significantly enhances the game development journey. Eclipse and IntelliJ IDEA stand out due to their comprehensive features that include code completion, debugging tools, and integrated libraries, crucial for developers.
Configuring Build Tools
In the Java ecosystem, Maven and Gradle are prominent for managing dependencies and automating tasks, thereby streamlining the development process.
```xml
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>game-lib</artifactId>
<version>1.0</version>
</dependency>
</dependencies>
```
The Maven `pom.xml` file snippet above declares a dependency on a hypothetical “game-lib” library, which Maven will automatically fetch and include in the project upon building.
Setting Up Game Libraries: How to Make a Game in Java?
Choosing the right game library is crucial, with options like LibGDX, LWJGL, and JMonkeyEngine being popular among developers. The decision should be based on the game’s requirements and the desired library integrated into the project’s IDE.
Establishing a robust Java environment is essential for beginning the game development journey. Proper tool and configuration selection is instrumental in navigating future challenges.
Choosing The Right Java Game Libraries
The selection of Java Game Libraries like LibGDX, LWJGL, or JMonkeyEngine is foundational to game development, influencing ease of development, performance, and game outcome.
LibGDX is celebrated for its versatility across platforms, LWJGL for its high-performance game development tools, and JMonkeyEngine for its 3D game development capabilities. The choice should align with the game’s needs, the developer’s experience level, and the target platform to facilitate a smoother development process.
Initializing Game Window And Canvas
The initial visual element of any game is its window, serving as the canvas for all game interactions. Proper setup ensures optimal performance and visual quality.
Java’s JFrame from the Swing library offers a straightforward approach to window creation, sufficient for many basic games. The integration of a Canvas element allows for drawing game visuals, while utilizing a BufferStrategy ensures smoother animations and graphics. Adjusting window properties to suit game needs is also crucial for an optimal gaming experience.
Implementing Game Loops
The game loop is the engine of a game, consistently updating the game state and rendering graphics to ensure smooth and responsive gameplay.
The basics of a game loop involve repetitively checking conditions, updating states, and rendering graphics. Implementing a fixed time step loop ensures consistent update rates across systems while handling input within the loop allows for real-time player interaction. Variable time step loops cater to games requiring precise updates, making the loop’s selection crucial for optimal game behavior and performance.
The foundation of game development hinges on the right setup, from the JDK installation and IDE selection to choosing build tools and game libraries. The initialization of the game window and canvas, coupled with the implementation of an appropriate game loop, sets the stage for bringing a game to life.
Engaging players through interactivity is a cornerstone of game design. Java offers a multitude of pathways for capturing and processing player inputs, each vital for crafting a dynamic and responsive gaming experience.
Handling User Input And Events
Incorporating user inputs, from simple keyboard strokes to complex joystick maneuvers, ensures players can interact seamlessly with the game environment.
Keyboard Input With KeyListener
Java utilizes the KeyListener interface for capturing keyboard actions, providing an avenue for real-time game control.
```java
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
public class Game implements KeyListener {
@Override
public void keyPressed(KeyEvent e) {
int keyCode = e.getKeyCode();
// Handle "UP" arrow key press
}
@Override
public void keyReleased(KeyEvent e) {
// Code for key release
}
@Override
public void keyTyped(KeyEvent e) {
// Code for key type
}
}
```
For instance, handling the pressing of the “UP” arrow key demonstrates how specific key actions can be identified and processed.
Mouse Input With MouseListener
For interactions requiring precision and nuance, such as clicks and cursor movements, Java employs MouseListener and MouseMotionListener interfaces.
```java
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.awt.event.MouseMotionListener;
public class Game implements MouseListener, MouseMotionListener {
@Override
public void mouseClicked(MouseEvent e) {
// Capture mouse click coordinates
}
@Override
public void mouseMoved(MouseEvent e) {
// Handle mouse movement
}
}
```
This setup enables the capture and utilization of mouse coordinates for interactive game mechanics.
Action Commands and Buttons
For games incorporating graphical user interfaces (GUIs), ActionListener facilitates the detection of button interactions, linking GUI elements with game logic.
```java
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
public class Game {
JButton startButton = new JButton("Start Game");
public Game() {
startButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// Invoke game logic on button press
}
});
}
}
```
Activating game functions through GUI elements like a “Start Game” button exemplifies the integration of user input into game flow.
Joystick and External Devices
To accommodate specialized control schemes, Java can incorporate external libraries like JInput, expanding input options to include devices such as joysticks and gamepads.
Capturing and processing player inputs forms the interactive core of the gaming experience, shaping the player’s journey and enhancing engagement with the game world.
Creating Sprites and Game Assets
Visual components and sound assets enrich the gaming environment, elevating the player’s immersive experience.
Loading Image Sprites
Java’s BufferedImage class facilitates the loading of image sprites, essential for visualizing characters, items, and more.
```java
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class Sprite {
private BufferedImage image;
public Sprite(String path) {
try {
image = ImageIO.read(new File(path));
} catch (IOException e) {
e.printStackTrace();
}
}
public BufferedImage getImage() {
return image;
}
}
```
Through this method, individual sprites can be loaded and displayed within the game.
Sprite Sheets and Animation
Animations often leverage sprite sheets, compact images containing multiple animation frames, to convey movement and action dynamically.
```java
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class AnimatedSprite {
private BufferedImage[] frames;
public AnimatedSprite(String path, int frameWidth, int frameHeight) {
// Logic to load and divide sprite sheet into frames
}
public BufferedImage getFrame(int index) {
return frames[index];
}
}
```
This approach efficiently manages animations by slicing sprite sheets into individual frames, facilitating smooth animated sequences.
Sound Assets with Clip
Complementing visuals with sound effects and music deepens the gameplay experience, using Java’s Clip interface to manage audio playback.
```java
import javax.sound.sampled.*;
public class GameSound {
private Clip clip;
public GameSound(String path) {
try {
// Load and play sound from path
} catch (Exception e) {
e.printStackTrace();
}
}
public void play() {
clip.start();
}
}
```
Integrating sound adds depth to the gaming environment, enhancing the overall ambiance and player immersion.
Building Game Logic and Mechanics
At the heart of every game lie its mechanics and logic, governing player interactions, movements, and the game’s core rules.
Character Movement
Implementing character movement is fundamental, often facilitated through event listeners that track keyboard inputs.
```java
import java.awt.event.KeyAdapter;
import java.awt
.event.KeyEvent;
public class PlayerMovement extends KeyAdapter {
// Logic to update movement based on key presses
}
```
This setup allows for the direct translation of user inputs into character movement, providing a responsive gameplay experience.
Collision Detection
Detecting interactions between game entities is crucial for dynamic environments, requiring mechanisms to determine when objects collide or intersect.
```java
public boolean isColliding(GameObject obj1, GameObject obj2) {
// Determine intersection between objects
}
```
Such functionality enables the game to respond to collisions, affecting gameplay through obstacles, item pickups, or enemy encounters.
Game State Management
Organizing gameplay into distinct states, such as starting, playing, and ending phases, aids in managing the game’s progression and user experience.
```java
public enum GameState {
START, PLAY, END;
}
public class GameManager {
// Logic to transition between game states
}
```
Through state management, games can offer structured experiences, guiding players from introduction to conclusion.
Implementing Power-Ups
Incorporating power-ups or boosts adds layers to the gameplay, offering temporary advantages or changes in game dynamics.
```java
public class PowerUp {
// Activate specific boosts or effects
}
```
Power-ups introduce strategic elements, encouraging players to seek advantages and enhancing the game’s depth and replayability.
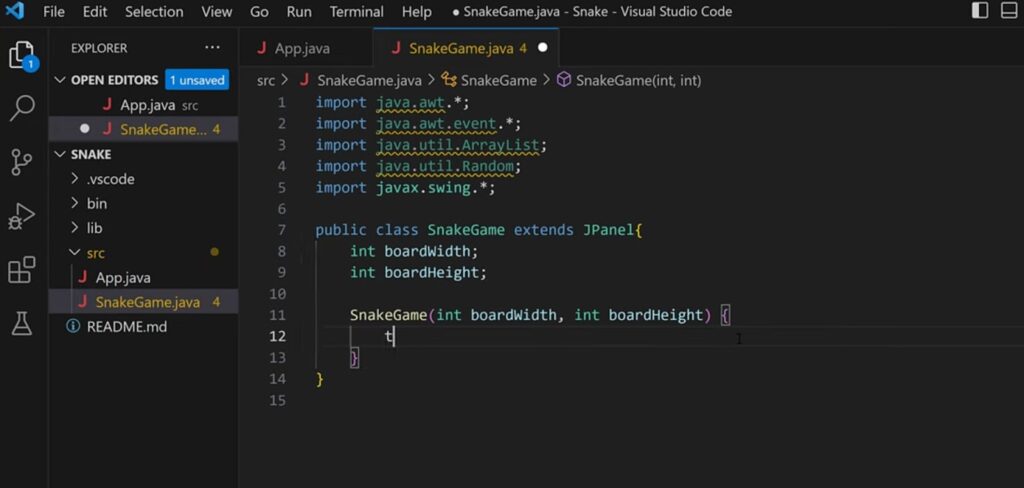
Implementing Sound And Music
Effective audio implementation, from impactful sound effects to engaging background music, plays a pivotal role in crafting memorable gaming experiences.
Playing Sound Effects
Sound effects for actions like jumping or collecting items provide immediate feedback, enriching player interaction.
```java
public void playSoundEffect(String path) {
// Play specific sound effect
}
```
Such auditory cues are essential for immersive gameplay, offering real-time response to player actions.
Background Music Loop
Continuous background music creates an atmosphere, setting the tone for the game environment.
```java
public void playBackgroundMusic(String path) {
// Loop background music continuously
}
```
This consistent auditory backdrop enhances the gaming atmosphere, contributing to the overall mood and setting.
Adjusting Volume
Allowing for volume control facilitates a customizable audio experience, catering to player preferences.
```java
public void setVolume(Clip clip, float volume) {
// Adjust volume level
}
```
Volume adjustment options ensure that sound levels are comfortable and enjoyable for the player, enhancing user experience.
Implementing comprehensive game mechanics and engaging audiovisual assets is essential for captivating gameplay. These elements work in tandem to create a rich, immersive world that entertains, challenges, and delights players.
Achieving fluid gameplay and mastering troubleshooting techniques are pivotal in the development of Java-based games, ensuring they not only captivate but also run seamlessly.
Optimizing Performance and Debugging
Memory Management
Java’s automatic memory management, courtesy of its garbage collector, significantly aids in managing resources. However, developers must still be vigilant about object creation to prevent unnecessary slowdowns.
```java
Player player = new Player();
for (int i = 0; i < 1000; i++) {
player = new Player();
}
```
In scenarios requiring multiple instances, it’s more resource-efficient to reuse objects whenever possible, minimizing the performance hit from excessive object instantiation.
Profiling Your Game
Utilizing profiling tools such as Java VisualVM or JProfiler is instrumental in detecting areas where performance may lag, by meticulously analyzing execution times and resource usage.
```java
long startTime = System.nanoTime();
// Game code execution
long endTime = System.nanoTime();
System.out.println("Execution time: " + (endTime - startTime) + " nanoseconds");
```
Employing this code snippet to measure the duration of specific operations helps in pinpointing inefficient code paths or operations.
Optimizing Graphics
Optimizing graphical output by limiting the number of active on-screen elements, using sprite sheets for animation, and minimizing transparency can lead to significant improvements in rendering speed and overall game performance.
Debugging Techniques
Java’s robust set of debugging tools aids developers in identifying and resolving issues within their code effectively.
Breakpoints And Step-Through Debugging
By setting breakpoints, developers can halt code execution at critical points to examine the state of the application, inspect variable values, and trace through the execution flow step-by-step.
```java
int result = computeScore(level, points);
```
Utilizing breakpoints on specific lines allows for a deeper investigation into how variables evolve over time and how the logic flows, facilitating the identification of problematic code segments.
Exception Handling
Efficiently managing exceptions not only clarifies the nature of errors but also prevents the application from terminating abruptly, ensuring a smoother user experience.
```java
try {
loadGameAssets();
} catch (Exception e) {
System.err.println("Error loading game assets: " + e.getMessage());
e.printStackTrace();
}
```
Capturing and logging exceptions gives developers a clear insight into the failures, enabling them to address and rectify issues more effectively.
Emphasizing regular performance checks, employing efficient memory management strategies, and adeptly navigating debugging tools are foundational practices in game development. These practices ensure that Java games not only engage but also provide a seamless and enjoyable experience for the user.
To Wrap Up
In conclusion, the journey of developing a game in Java encompasses much more than just crafting engaging stories and beautiful graphics. It demands a meticulous approach towards optimizing performance and a strategic plan for debugging. The practices of efficient memory management, rigorous profiling to identify and resolve bottlenecks, graphical optimizations to ensure smooth rendering, and adept use of debugging techniques form the backbone of high-quality game development. Employing these techniques not only enhances the gameplay experience but also significantly reduces the likelihood of performance issues and bugs that can detract from the player’s enjoyment.
Moreover, the strategic handling of exceptions and the insightful use of breakpoints and step-through debugging illuminate the path to understanding and rectifying the root causes of issues. This comprehensive approach ensures that the game not only meets the developers’ vision but also exceeds players’ expectations in terms of reliability and responsiveness. Ultimately, embracing these practices elevates the standard of game development, leading to creations that are not only technically robust but also widely appreciated for their smooth and immersive gameplay experiences.