XML (Extensible Markup Language) is a widely-used format for storing and transmitting data. It is commonly used in web development, as well as in other industries such as finance and healthcare. In order to access and manipulate XML data, developers use parsers, which are software components that read and interpret the XML code. One of the most popular and efficient parsers for Java is the Simple API for XML (SAX). In this tutorial, we will discuss how to use the SAX parser to read XML files in Java.
Prerequisites
Before we dive into the details of using the SAX parser, there are a few prerequisites that you need to have in place. First and foremost, you will need to have the Java Development Kit (JDK) installed on your system. The JDK includes the Java Runtime Environment (JRE), which is necessary for running Java applications. Additionally, you will need a text editor or an integrated development environment (IDE) such as Eclipse or NetBeans to write and run your Java code.
Giving the following XML document
In order to demonstrate how to read XML files using the SAX parser, we will be using a sample XML document. You can either create your own XML file or use the one provided below:
Planning
2 weeks
Development
4 weeks
Testing
2 weeks
Save this XML document to your local disk for later use.
How to Read XML file via SAX Parser in Java?
Now that we have our prerequisites in place and a sample XML document to work with, let’s dive into the steps for reading XML files using the SAX parser in Java.
Step 1: Create a class that extends DefaultHandler
The first step is to create a Java class that extends the DefaultHandler class. This class provides default implementations for all the methods defined in the ContentHandler, DTDHandler, EntityResolver, and ErrorHandler interfaces. These methods are used by the SAX parser to handle different types of events that occur while parsing an XML document.
Step 2: Override the necessary methods
There are five methods defined in the DefaultHandler class that you may need to override in order to handle specific events during the parsing process. These methods are:
- startDocument(): This method is called at the beginning of the parsing process;
- endDocument(): This method is called at the end of the parsing process;
- startElement(String uri, String localName, String qName, Attributes attributes): This method is called when the parser encounters the start of an element in the XML document;
- endElement(String uri, String localName, String qName): This method is called when the parser encounters the end of an element in the XML document;
- characters(char[] ch, int start, int length): This method is called when the parser encounters character data within an element.
You can override these methods in your class to perform custom actions based on the events that occur during the parsing process.
Step 3: Create the corresponding Java bean
In order to store the data from the XML document, we need to create a Java bean that represents the structure of the XML elements. In our example, we will create a Phase class with attributes for the phase ID, name, and duration.
Step 4: The code to Read XML file via SAX Parser in Java
Now that we have our class and bean set up, we can write the code to read the XML document using the SAX parser. The following code snippet shows how this can be done:
// Create an instance of the SAXParserFactory
SAXParserFactory factory = SAXParserFactory.newInstance();
// Create an instance of the SAXParser
SAXParser saxParser = factory.newSAXParser();
// Create an instance of your custom handler class
CustomHandler handler = new CustomHandler();
// Parse the XML document using the handler
saxParser.parse(“path/to/xml/file”, handler);
// Get the list of phases from the handler
List phases = handler.getPhases();
In this code, we first create an instance of the SAXParserFactory class, which is responsible for creating instances of the SAXParser class. We then use the SAXParser to parse the XML document, passing in the path to the file and an instance of our custom handler class. Finally, we can retrieve the list of phases from the handler and use them as needed.
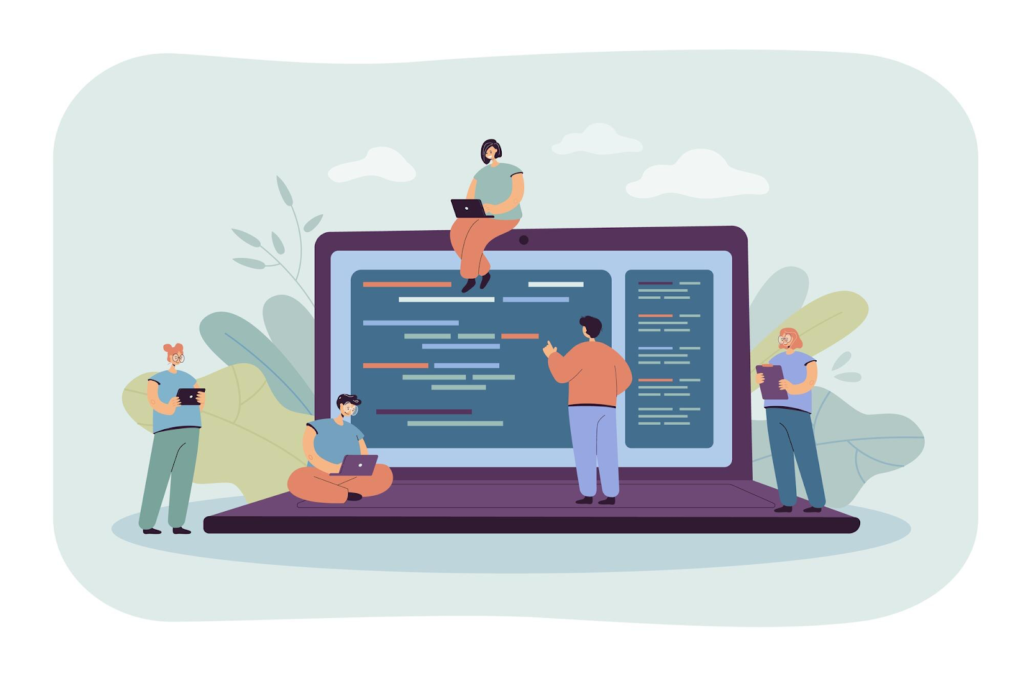
Output after parsing XML via SAX
After running the code above, we should have a list of Phase objects containing the data from our XML document. We can then use this data to perform any necessary operations or display it in a user interface. For example, we could print out the names and durations of each phase in the list:
for (Phase phase : phases) {
System.out.println(phase.getName() + ": " + phase.getDuration());
}
This would produce the following output:
Planning: 2 weeks
Development: 4 weeks
Testing: 2 weeks
Conclusion
In this tutorial, we have discussed how to read XML files using the SAX parser in Java. We first covered the prerequisites for using the SAX parser, including having the JDK and a text editor or IDE installed. We then provided a sample XML document for testing purposes. Next, we went through the steps for reading XML files using the SAX parser, which involved creating a custom handler class, overriding necessary methods, and creating a corresponding Java bean. Finally, we showed how to retrieve and use the data from the XML document after parsing it with the SAX parser. With this knowledge, you should now be able to efficiently read and manipulate XML data in your Java applications.