CXF, short for Common XML Framework, is an open-source web services framework that provides a robust and easy-to-use platform for building SOAP-based and RESTful web services. It is widely used in enterprise applications due to its support for a variety of protocols and data formats, as well as its compatibility with different programming languages. In this article, we will focus on one of the key components of CXF – the CXF client – and explore its features, benefits, and how to use it effectively.
What is a CXF Client?
A CXF client is a Java-based API that allows developers to interact with services implemented using the CXF framework. It enables applications to communicate over different protocols, such as HTTP, HTTPS, JMS, and many more, by providing a high-level abstraction layer. This means that developers do not have to worry about the underlying transport details, making it easier to build web service clients.
Benefits of Using a CXF Client
- Protocol Independence: One of the main advantages of using a CXF client is its ability to work with various protocols, including SOAP, REST, and CORBA. This gives developers the freedom to choose the most suitable protocol for their application without having to change any code;
- Flexibility: CXF clients offer a flexible approach to building web service clients. It supports both programmatic and declarative styles of coding, giving developers the flexibility to choose the approach that best suits their needs;
- Wide Language Support: CXF has excellent support for multiple programming languages such as Java, C++, and JavaScript, making it a popular choice among developers;
- Interoperability: CXF clients are designed to work seamlessly with other web service frameworks, ensuring interoperability with other systems;
- Security: CXF provides built-in support for various security mechanisms, including WS-Security and OAuth, making it a secure choice for building web service clients;
- Monitoring and Management: CXF comes with features that allow developers to monitor and manage their web services effectively using tools such as Apache Camel and Apache Karaf.
How to Use a CXF Client
Now that we understand the benefits of using a CXF client let’s dive into the details of how to use it in your applications. The following sections will guide you step by step on how to get started with CXF client.
Setting Up Your Development Environment
To start using the CXF client, you need to set up your development environment. This includes installing Java and Maven, which are essential for building and running CXF projects. Here are the steps to follow:
Installing Java
- Download and install the latest JDK (Java Development Kit) from Oracle’s website;
- Set up the JAVA_HOME environment variable to point to the location where you installed Java;
- Add the Java bin directory to your PATH variable.
Installing Maven
- Download the latest version of Maven from the official website;
- Unzip the downloaded file to a desired location on your computer;
- Set up the MAVEN_HOME environment variable to point to the location where you extracted Maven;
- Add the Maven bin directory to your PATH variable.
Creating a CXF Project
Once your development environment is set up, you can create your first CXF project using Maven. Follow these steps:
- Open your terminal/command prompt and navigate to the directory where you want to create your project;
- Run the following command: mvn archetype:generate -B -DarchetypeGroupId=org.apache.cxf.archetype -DgroupId=com.example -DartifactId=my-cxf-project -Dversion=1.0-SNAPSHOT -DarchetypeArtifactId=cxf-jaxws-javafirst;
- This will generate a CXF project with the necessary files and dependencies for creating a web service client. You can change the values of groupId, artifactId, and version according to your project requirements;
- Navigate into the generated project directory (my-cxf-project) and open it in your preferred IDE.
Writing Your First CXF Client
Now that you have set up your project let’s see how to write a basic CXF client to consume a web service. For this example, we will use the Calculator Service provided by Silicon Valley Code Camp.
Create a Java Class
- In your IDE, create a new Java class named CalculatorClient;
- Add the following code to the class:
package com.example;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
import org.apache.cxf.interceptor.LoggingInInterceptor;
import org.apache.cxf.interceptor.LoggingOutInterceptor;
import org.apache.cxf.calculator.Calculator;
import org.apache.cxf.calculator.CalculatorSoap;
public class CalculatorClient {
public static void main(String[] args) {
// Set up the proxy factory bean
JaxWsProxyFactoryBean proxyFactory = new JaxWsProxyFactoryBean();
// Configure the endpoint URL
proxyFactory.setAddress("https://www.siliconvalley-codecamp.com/Calculatorservice/services/Calculator?wsdl");
// Set the service interface
proxyFactory.setServiceClass(Calculator.class);
// Add logging interceptors for debugging
proxyFactory.getInInterceptors().add(new LoggingInInterceptor());
proxyFactory.getOutInterceptors().add(new LoggingOutInterceptor());
// Create the client
Calculator client = (Calculator) proxyFactory.create();
// Invoke the add method and print the result
System.out.println("5 + 3 = " + client.add(5, 3));
}
}
- Make sure to replace the endpoint URL with the correct one for your service;
- Run the main method in the CalculatorClient class.
Understanding the Code
Let’s break down the code we just wrote to understand how it works:
- First, we import the necessary classes from the CXF library;
- Then, we create a JaxWsProxyFactoryBean object to act as our client’s proxy factory;
- Next, we set the endpoint address of our web service using the setAddress method;
- We also specify the service interface that our client will use to communicate with the service using the setServiceClass method;
- To help with debugging, we add logging interceptors to the proxy factory using the getInInterceptors and getOutInterceptors methods;
- Finally, we create an instance of our client using the create method and invoke the add method on it to perform the addition operation.
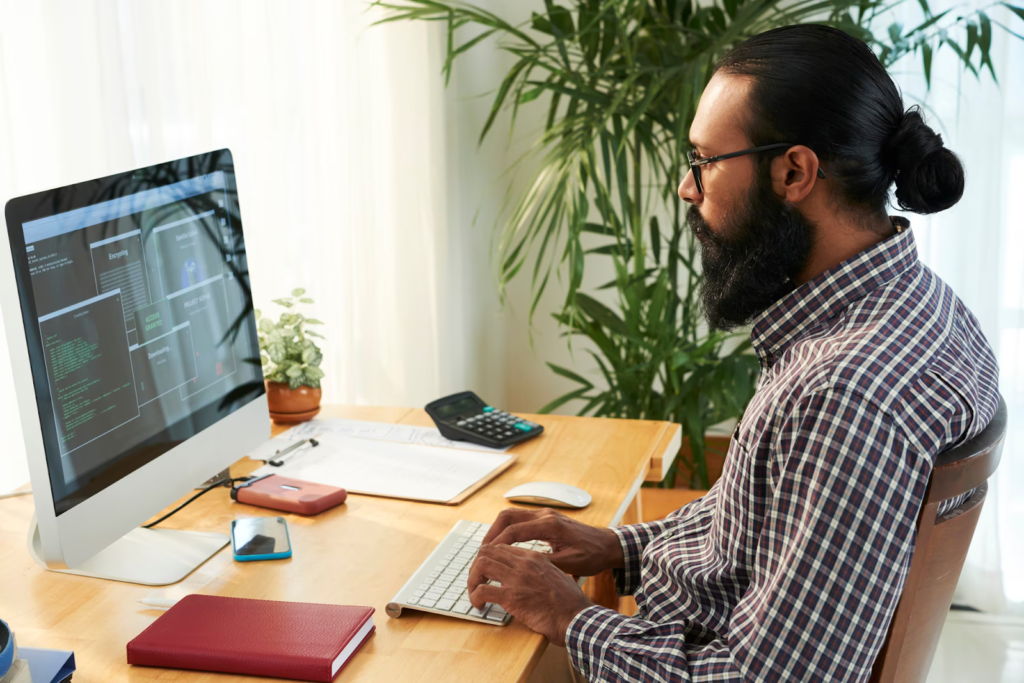
Advanced Features of CXF Client
CXF client offers several advanced features that can be used to enhance the functionality and performance of your application. Let’s dive into some of these features in more detail.
WSDL-to-Java Mapping Tool
CXF provides a tool called wsdl2java that generates Java code from a WSDL (Web Services Description Language) file. This eliminates the need to manually write the client-side code, saving time and effort. The generated code includes all the necessary classes and interfaces needed to access the web service.
To use this tool, follow these steps:
- Open your terminal/command prompt and navigate to the project directory;
- Run the following command: mvn org.apache.cxf:cxf-codegen-plugin:wsdl2java -DwsdlRoot=$ with the package name you want to use for the generated code;
- This will generate Java classes in the specified package that can be used to interact with the web service.
Using the CXF Client API
CXF client provides a powerful API that can be used to customize the behavior of your web service clients. Some common use cases include configuring timeouts and connection pooling, handling exceptions, and setting up message interceptors. Here’s an example of how to use the API to set a timeout for your client:
ClientProxy.getClient(client).getConduit().close(5000);
This code closes the client’s HTTP connection after 5 seconds if the connection is idle. You can use similar methods to configure other settings for your client.
Asynchronous Communication
CXF client also supports asynchronous communication, where the client can send a request and continue with other tasks while waiting for the response from the service. This is useful for applications that need to process multiple requests simultaneously without blocking the main thread. To enable asynchronous communication, follow these steps:
- Add the javax.xml.ws.AsyncHandler interface to your service interface;
- Implement the handleResponse method to handle the response from the service asynchronously;
- Invoke the service method using the asyncInvoke method instead of the regular invoke method.
An example of how to implement this is shown below:
public class CalculatorClient {
public static void main(String[] args) {
JaxWsProxyFactoryBean proxyFactory = new JaxWsProxyFactoryBean();
// Configure the endpoint URL and service interface
// ...
// Create the client
Calculator client = (Calculator) proxyFactory.create();
// Create an instance of the AsyncHandler interface
AsyncHandler handler = new AsyncHandler() {
@Override
public void handleResponse(Response future) {
try {
AddResponse response = future.get();
System.out.println("5 + 3 = " + response.getAddResult());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
};
// Invoke the add method asynchronously
Future future = client.addAsync(5, 3, handler);
// Do other tasks while waiting for the response
// ...
}
}
Using CXF with Spring
CXF integrates well with Spring to provide a more flexible and configurable way of building web service clients. To use CXF with Spring, you can either configure it using XML or annotations. Here’s an example of how to configure a CXF client using annotations:
@Configuration
public class AppConfig {
@Bean
public Calculator calculator() {
// Set up the proxy factory bean
JaxWsProxyFactoryBean proxyFactory = new JaxWsProxyFactoryBean();
// Configure the endpoint URL
proxyFactory.setAddress("https://www.siliconvalley-codecamp.com/Calculatorservice/services/Calculator?wsdl");
// Set the service interface
proxyFactory.setServiceClass(Calculator.class);
// Add logging interceptors for debugging
proxyFactory.getInInterceptors().add(new LoggingInInterceptor());
proxyFactory.getOutInterceptors().add(new LoggingOutInterceptor());
// Create the client
return (Calculator) proxyFactory.create();
}
}
Then, you can simply autowire the Calculator bean in your application and use it to access the web service.
Conclusion
In this article, we have covered the basics of CXF client, its benefits, and how to use it effectively in your applications. We also explored some advanced features that can enhance the functionality and performance of your web service clients. With its support for multiple protocols, flexible programming styles, and compatibility with other frameworks, CXF client is a reliable choice for building robust and secure web service clients. Whether you are a beginner or an experienced developer, CXF client offers a user-friendly and efficient way to interact with web services. So go ahead, give it a try, and see how it can improve your web service development experience.