Mastering the art of printing strings in Java marks one of the fundamental skills every novice Java developer embarks upon. Whether you seek to convey a message to the user or debug your code, the proficiency in effectively printing strings remains paramount. This comprehensive guide is tailored to navigate you through diverse methodologies of string printing in Java, addressing assorted scenarios and individual preferences.
Mastering System.out.println(): A Comprehensive Guide to Printing Text in Java
Printing text in Java is a fundamental operation, and one of the simplest ways to achieve this is by utilizing the System.out.println() method. This method, belonging to the System class, serves as a reliable means to showcase output directly onto the console, making it a go-to choice for developers seeking to communicate with their programs.
Let’s delve deeper into how this method works and its significance:
How System.out.println() Works:
When you use System.out.println(), you’re essentially instructing Java to display the specified text onto the console. Here’s a breakdown of its functionality:
Invocation: To employ System.out.println(), simply type the method name followed by the string you wish to print within parentheses.
Syntax Example:
System.out.println("Hello, world!");
Output: The specified text will be showcased on the console.
Utilizing System.out.println() in Code:
Below is a snippet demonstrating the application of System.out.println() within a Java program:
public class HelloWorld {
public static void main(String[] args) {
String message = "Hello, world!";
System.out.println(message);
}
}
Understanding the Output:
Upon executing the above code, the output will be:
Hello, world!
Mastering Output Control in Java: The Power of System.out.print()
In Java programming, when you need to display a string without automatically moving to the next line, you can employ the System.out.print() method. Unlike its counterpart println(), which automatically appends a newline character, print() simply outputs the text without any line break. This can be particularly useful in scenarios where you want to format your output precisely or concatenate multiple strings without intervening line breaks.
Here’s a detailed breakdown of how to use System.out.print() effectively:
Syntax:
System.out.print(string);
Example:
public class HelloWorld {
public static void main(String[] args) {
String message = "Hello, ";
System.out.print(message);
System.out.println("world!");
}
}
Output:
Hello, world!
Key Points to Note:
- No Automatic Line Break: Unlike println(), print() does not automatically move the cursor to the next line after displaying the string. This allows for more control over the output format;
- Concatenation: You can concatenate multiple strings using print() without introducing line breaks between them. This is useful for building complex output formats or generating dynamic messages;
- Formatting Control: Since print() doesn’t add a newline character, you can precisely control the layout of your output, ensuring it appears exactly as desired;
- Usage in Loops: When iterating through loops or processing large datasets, using print() can result in more compact and readable output, especially when dealing with incremental updates or progress indicators;
- Combining with println(): There may be cases where you want to mix both print() and println() methods for specific formatting requirements. Experimenting with different combinations can help achieve the desired output structure;
- Debugging Purposes: During debugging, print() can be invaluable for selectively displaying variable values or tracing program flow without cluttering the console with unnecessary line breaks.
Mastering Formatted String Printing in Java with PrintStream.printf()
Java offers a convenient method for formatted string printing known as PrintStream.printf(). This feature enables you to embed values into a string using format specifiers, reminiscent of the printf function in the C programming language. Incorporating this method into your Java code not only enhances readability but also provides a structured approach to presenting data.
Here’s an illustrative example to demonstrate how PrintStream.printf() works:
public class FormatExample {
public static void main(String[] args) {
String name = "John";
int age = 30;
double salary = 50000.50;
System.out.printf("Name: %s, Age: %d, Salary: %.2f", name, age, salary);
}
}
Upon executing the code, the output will be:
Name: John, Age: 30, Salary: 50000.50
Let’s delve deeper into the format string used in the printf() method:
- %s: This serves as a placeholder for a string;
- %d: Represents a placeholder for an integer;
- %.2f: This acts as a placeholder for a floating-point number with two decimal places.
Utilizing these placeholders ensures that the values provided after the format string are correctly interpreted and displayed in the desired format.
Advantages of Using PrintStream.printf():
- Readability Enhancement: By incorporating formatted strings, your code becomes more comprehensible as it explicitly defines the structure of the output;
- Flexible Formatting: PrintStream.printf() allows for precise formatting of various data types, enabling customization according to specific requirements;
- Concise Syntax: With format specifiers, you can achieve complex string constructions with minimal code, promoting cleaner and more maintainable codebases;
- Error Prevention: Using format specifiers reduces the likelihood of errors in string formatting, ensuring accurate representation of data;
- Consistency: Adopting PrintStream.printf() ensures consistency in the presentation of output across different parts of your application, enhancing user experience and usability.
Troubleshooting Techniques and Insights
Mastering the art of troubleshooting printing issues in Java is pivotal for every programmer navigating the intricacies of this versatile language. Even the seemingly straightforward task of printing a string can morph into a labyrinth of syntax errors, runtime glitches, or logical snags. Let’s delve into a treasure trove of techniques to unearth solutions when Java printing woes rear their heads:
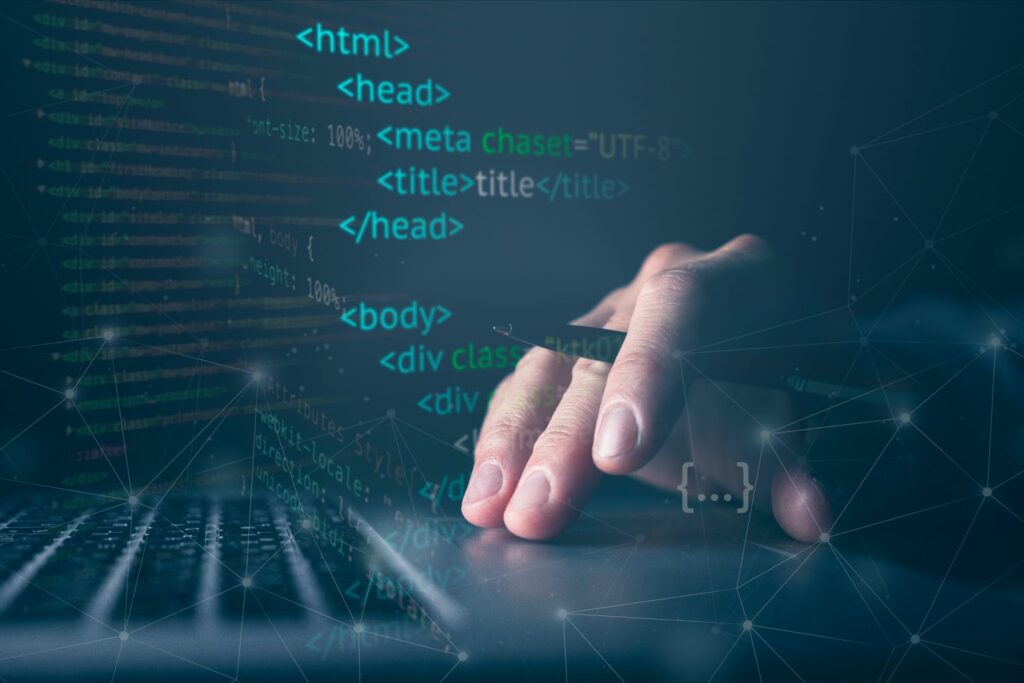
Syntax Errors Demystified
Syntax errors serve as the watchdogs of the Java language, vigilantly flagging any violations of its grammatical rules. These pesky errors, often apprehended by the compiler, can swiftly throw a spanner in the works of your code execution. When grappling with syntax-related printing hiccups, keep an eagle eye out for:
- Missing Semicolons: Check for proper termination of statements;
- Mismatched Parentheses, Braces, or Brackets: Ensure balance in your code’s structure;
- Typo Tyranny: Beware of sneaky spelling errors in variable names or method invocations;
- Quotation Quagmires: Double-check the integrity of your quotation marks, particularly when handling string literals.
Resolving syntax errors mandates a meticulous dissection of your code, aligning it harmoniously with the syntax paradigms laid out by Java.
Decoding Runtime Exceptions
Runtime exceptions are the covert agents of chaos, stealthily lurking within your program’s execution and wreaking havoc when least expected. These exceptions, when perturbing string printing operations, often manifest as:
- NullPointerException: Stealthily surfaces when attempting to print a null reference;
- ArrayIndexOutOfBoundsException: Emerges from the shadows when venturing beyond the confines of array bounds;
- NumberFormatException: Sneaks into the fray upon stumbling while parsing strings into numeric values.
To crack the code of runtime exceptions, decipher the cryptic stack traces furnished by the Java Virtual Machine (JVM). Equip yourself with debugging artillery like System.out.println() statements or wield a debugger to trace the convoluted pathways of your program and pinpoint the origins of these unruly exceptions.
Mastering Logic Errors in Program Execution
Logic errors can be a tricky adversary in software development, often lurking beneath the surface and manifesting as incorrect outputs without raising any immediate red flags. Uncovering and addressing these elusive bugs is essential for ensuring the reliability and accuracy of your programs. Here’s how you can effectively tackle logic errors, especially when dealing with printing strings:
- Review Code Logic: Take a deep dive into your code’s logic to ensure it comprehensively handles all possible scenarios. Break down complex algorithms and conditional statements to pinpoint potential areas of logical inconsistency;
- Watch Out for Off-by-One Errors: One of the most common culprits behind logic errors is the notorious off-by-one mistake. Double-check loop conditions and array indices to ensure they are correctly set up to iterate over the desired range;
- Verify Variable Values: Before printing any strings, it’s crucial to verify that the variables involved contain the expected values. Debugging statements or using specialized debugging tools can help you track the flow of execution and identify discrepancies in variable assignments;
- Utilize Logging and Debugging Tools: Incorporate logging statements strategically throughout your code to trace the execution flow and capture the values of critical variables at various stages. Additionally, leverage debugging tools provided by your IDE to step through the code and analyze its behavior in real-time;
- Test with Different Inputs: Comprehensive testing is key to uncovering hidden logic errors. Experiment with a variety of inputs, including edge cases and boundary conditions, to ensure that your program behaves as expected under diverse scenarios.
By adopting these strategies, you can enhance your ability to detect and rectify logic errors, ultimately bolstering the reliability and robustness of your software solutions.
Navigating Encoding and Character Challenges
In the realm of string manipulation, encountering non-ASCII characters or special symbols is not uncommon. However, handling these elements gracefully requires attention to encoding and character issues. Here’s how you can navigate this terrain effectively:
- Choose Compatible Character Encoding: Ensure that your Java source files are encoded using a character encoding scheme that supports a wide range of characters, such as UTF-8. This ensures seamless handling of diverse character sets without loss of information;
- Employ Escape Sequences for Unicode Characters: When dealing with Unicode characters that cannot be directly represented in your source code, utilize escape sequences (\uXXXX) within string literals to specify these characters explicitly. This ensures that the intended characters are accurately preserved during compilation and execution;
- Test Across Multiple Environments: Validate the behavior of your string processing routines across different environments to ensure compatibility and consistency. This includes testing on various operating systems and platforms to uncover any potential encoding-related discrepancies.
By adhering to these guidelines, you can mitigate encoding and character issues effectively, enabling smooth handling of diverse textual data within your Java applications.
Optimizing Environment and Configuration for Seamless Printing
Printing issues can often stem from environmental or configuration factors, necessitating meticulous attention to the setup of your development environment. Here’s how you can optimize your environment and configuration settings to ensure seamless printing functionality:
- Verify JDK Installation and Version: Confirm that the Java Development Kit (JDK) is installed correctly on your system and is up-to-date with the latest patches and updates. This ensures compatibility with the latest language features and bug fixes;
- Check Runtime Environment Configuration: Ensure that your runtime environment is configured appropriately, with all necessary dependencies and classpath entries properly specified. This prevents runtime errors related to missing libraries or conflicting configurations;
- Monitor System Resources: Keep an eye on system resource utilization, such as memory and CPU usage, especially when dealing with large-scale printing operations. Optimizing resource allocation can prevent performance bottlenecks and ensure smooth execution of printing tasks;
- Consider Platform-specific Considerations: Take into account any platform-specific nuances or limitations that may impact printing functionality, particularly when deploying your application across diverse operating systems or hardware configurations.
Conclusion
Mastering the art of string printing in Java is quintessential for any programmer traversing the realm of programming. Within the Java domain, a plethora of techniques exists to cater to your diverse printing needs, whether you’re conveying succinct messages or intricately formatting output. Familiarizing yourself with the intricacies of System.out.println(), System.out.print(), and PrintStream.printf() empowers you to navigate through an array of printing tasks seamlessly within your Java projects. Delve into experimentation with these methods, seamlessly integrating them into your codebase, thereby elevating the readability and user experience of your applications to unprecedented heights.