JSON, or JavaScript Object Notation, has emerged as a widely embraced data exchange format, prized for its straightforwardness and adaptability. Within the realm of Java programming, handling JSON data parsing stands out as a frequent necessity, particularly in scenarios involving web APIs or configuration data storage. This article embarks on an exploration of diverse techniques for JSON parsing in Java, meticulously tailored to newcomers while offering illuminating perspectives on varied methodologies.
Exploring JSON Data: A Beginner’s Guide
JSON (JavaScript Object Notation) serves as a versatile data format, facilitating easy comprehension and manipulation. Let’s delve into this essential concept with a practical example:
{
"name": "John Doe",
"age": 30,
"city": "New York"
}
In this JSON snippet, we encounter a trio of key-value pairs: name, age, and city. Each key serves as an identifier, while the accompanying values can vary from strings and numbers to booleans, arrays, or even nested JSON objects. JSON’s simplicity and flexibility render it ubiquitous across various domains, from web development to data interchange protocols.
Key Features of JSON:
- Lightweight: JSON boasts a minimalist syntax, making it concise and easily readable by both humans and machines;
- Platform Agnostic: Compatible with virtually all programming languages, JSON facilitates seamless data exchange across different platforms and systems;
- Extensible: JSON supports nesting of data structures, enabling the representation of complex hierarchical relationships with ease;
- Human-Readable: The structured format of JSON enhances its readability, aiding in debugging and troubleshooting processes.
Navigating JSON in Java: Leveraging Powerful Libraries
Java, being a widely-used programming language, offers robust libraries for JSON processing, simplifying data parsing and manipulation tasks. Here are some noteworthy options:
- Jackson: Developed by FasterXML, Jackson stands out as a premier choice for JSON parsing and serialization/deserialization tasks in Java. Its high performance and extensive feature set make it a preferred solution for developers across various domains;
- Gson: Hailing from the arsenal of Google, Gson emerges as another formidable contender in the realm of JSON processing. Renowned for its simplicity and efficiency, Gson facilitates seamless conversion between Java objects and JSON representations, streamlining data interchange workflows;
- org.json: As an integral component of the Java EE specification, org.json offers a straightforward approach to working with JSON data. While relatively lightweight, it provides essential functionalities for parsing and manipulating JSON structures, catering to diverse use cases within the Java ecosystem.
Exploring JSON Parsing with org.json Library
If you’re delving into JSON parsing, especially with Java, the org.json library offers a straightforward approach. Below, we’ll guide you through the process of parsing JSON using this library and highlight some essential steps and tips for seamless integration.
Adding Dependency
Before diving into JSON parsing with org.json, ensure that you’ve added the necessary dependency to your project. If you’re utilizing Maven for dependency management, follow these steps:
Open your project’s pom.xml file. Insert the following dependency:
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20210307</version>
</dependency>
By including this dependency, you ensure that your project has access to the org.json library for parsing JSON data effortlessly.
Parsing JSON Data
With the dependency set up, parsing JSON becomes a breeze. Below, we’ll outline the process step by step:
- Import org.json.JSONObject: Begin by importing the JSONObject class from the org.json package. This class provides functionalities for handling JSON objects in Java;
- Create JSON String: Prepare your JSON data in the form of a string. This string will later be parsed into a JSONObject instance;
- Instantiate JSONObject: Utilize the JSONObject class to parse the JSON string created in the previous step. This instantiation enables you to access and manipulate the JSON data conveniently.
Example Implementation
Here’s a simple example demonstrating JSON parsing using org.json:
import org.json.JSONObject;
public class JsonParserExample {
public static void main(String[] args) {
// JSON string to be parsed
String jsonString = "{\"name\":\"John Doe\",\"age\":30,\"city\":\"New York\"}";
// Parsing JSON string into JSONObject
JSONObject jsonObject = new JSONObject(jsonString);
// Accessing values
String name = jsonObject.getString("name");
int age = jsonObject.getInt("age");
String city = jsonObject.getString("city");
// Printing values
System.out.println("Name: " + name);
System.out.println("Age: " + age);
System.out.println("City: " + city);
}
}
Exploring JSON Parsing with Jackson and Gson Libraries
JSON parsing is a crucial aspect of modern software development, allowing seamless interchange of data between applications. Among the many libraries available for Java, Jackson and Gson stand out as popular choices. While they offer similar functionality, understanding their nuances can greatly benefit developers. Let’s delve into parsing JSON using Jackson, followed by Gson.
Parsing JSON with Jackson
Jackson provides a robust framework for working with JSON in Java applications. Here’s a basic example showcasing its usage:
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonParserExample {
public static void main(String[] args) throws Exception {
String jsonString = "{\"name\":\"John Doe\",\"age\":30,\"city\":\"New York\"}";
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
// Accessing values
String name = jsonNode.get("name").asText();
int age = jsonNode.get("age").asInt();
String city = jsonNode.get("city").asText();
// Printing values
System.out.println("Name: " + name);
System.out.println("Age: " + age);
System.out.println("City: " + city);
}
}
Exploration and Explanation
- Importing Necessary Packages: Before delving into JSON parsing, ensure you’ve imported the required packages from the Jackson library;
- Reading JSON Data: Jackson’s ObjectMapper is the cornerstone for parsing JSON. It efficiently converts JSON strings into a JsonNode object, providing access to JSON elements;
- Accessing JSON Values: Utilizing JsonNode, developers can access JSON values seamlessly. In our example, we extract the name, age, and city fields from the JSON object;
- Printing Extracted Values: Demonstrating the parsed data, we print out the extracted name, age, and city to the console;
- Error Handling: Remember to handle exceptions appropriately, especially when parsing JSON strings. In real-world scenarios, input data may not always conform to expected formats.
Troubleshooting JSON Parsing Issues in Java
Parsing JSON in Java is a fundamental task in many applications, but it’s not always smooth sailing. Errors and unexpected behaviors can crop up, particularly for those new to the process. Understanding common issues and knowing how to troubleshoot them is crucial for efficient development. Below are comprehensive tips and insights for addressing JSON parsing problems in Java.
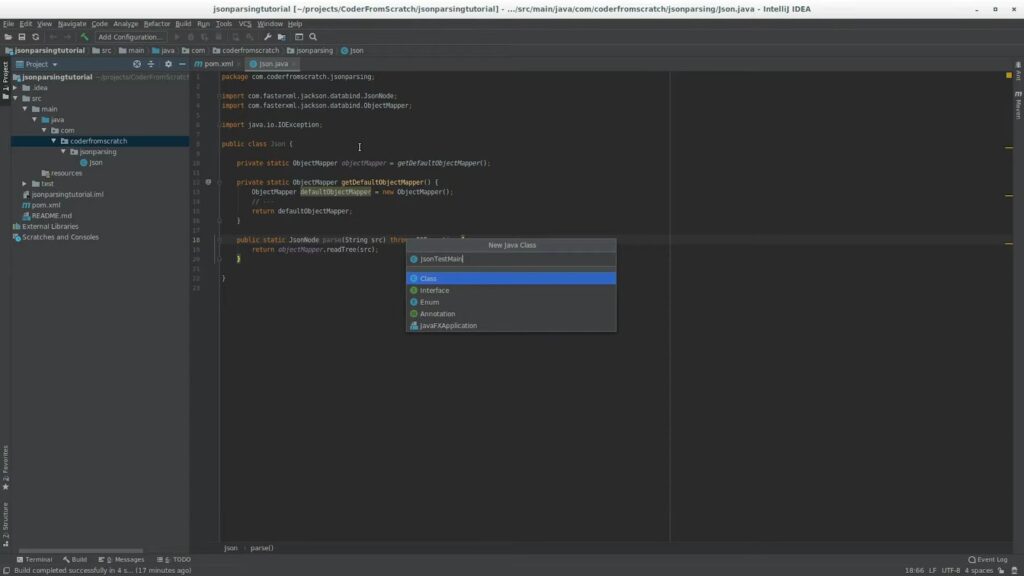
1. Validating JSON Format
Ensuring that your JSON data is properly formatted is the first step in troubleshooting parsing issues. Even minor syntax errors can cause parsing failures, so meticulous validation is key. Here’s how to tackle it:
- Utilize online JSON validation tools or IDE plugins dedicated to JSON formatting. These tools can quickly highlight syntax errors and inconsistencies;
- Verify that your JSON adheres to the standard format, including correct placement of commas, braces, and quotation marks;
- Check for common mistakes such as missing or extra commas, mismatched braces, or incorrect nesting.
2. Navigating Library Compatibility Challenges
When integrating third-party JSON parsing libraries like Jackson or Gson into your Java project, ensuring compatibility is paramount. Outdated or mismatched library versions can lead to a slew of unexpected errors or even outright failures. Here’s how to navigate library compatibility issues effectively:
- Check Java Environment Compatibility: Before integrating a JSON parsing library, verify that it’s compatible with your Java environment. This includes considering factors such as JDK version and any dependencies required by the library;
- Refer to Library Documentation: Each library typically provides detailed documentation outlining its compatibility matrix. Consult this documentation to ensure you’re using the correct version that aligns with your project’s requirements;
- Stay Updated: Keep abreast of updates and releases for your chosen library. Updates often include bug fixes, performance improvements, and enhanced compatibility with newer Java versions;
- Test Rigorously: Prior to deployment, thoroughly test your application with the chosen library version. This includes running compatibility tests across different Java environments and scenarios to catch any potential compatibility issues early on.
3. Unraveling Encoding and Character Set Conundrums
Properly encoding JSON data is crucial to prevent character encoding mishaps that can render your data unreadable or garbled. Here’s a comprehensive approach to tackle encoding and character set issues:
- UTF-8 Encoding: Encode your JSON data using UTF-8 to ensure universal compatibility across various platforms and systems. This encoding scheme supports a vast range of characters, including those from different languages and scripts;
- Validate Encoding Consistency: Confirm that both the JSON data and your Java application are using the same encoding scheme, preferably UTF-8. Mismatched encoding can lead to data corruption or misinterpretation;
- Handle Special Characters: Be mindful of special characters that may require escaping or special handling, especially in string values within the JSON data. Failure to properly handle such characters can result in parsing errors or unexpected behavior;
- Use Encoding Detection Tools: Employ tools or libraries capable of detecting the encoding of your JSON data automatically. These tools can help identify encoding discrepancies and facilitate resolution;
- Consider Data Sources: If your JSON data originates from external sources, ensure that the encoding is explicitly stated or standardized to prevent ambiguity during parsing.
4. Mastering Logging and Debugging Techniques
Effective logging and debugging are indispensable tools for unraveling parsing errors and tracing code flow. Here’s how to leverage these techniques for efficient troubleshooting:
- Strategic Logging: Incorporate logging statements strategically throughout your codebase to track the execution flow and capture relevant information. Include details such as JSON input, method parameters, and intermediate variables to aid in diagnosis;
- Utilize Debugging Tools: Leverage integrated development environments (IDEs) like IntelliJ IDEA or Eclipse, which offer robust debugging features. Set breakpoints, inspect variables, and step through code execution to pinpoint parsing errors with precision;
- Enable Logging Levels: Configure logging frameworks to utilize different log levels (e.g., debug, info, error) based on the severity of parsing issues. This allows for fine-grained control over the verbosity of log output, aiding in diagnosis without overwhelming logs;
- Collaborative Debugging: Encourage collaborative debugging sessions where team members can share insights and approaches to resolving parsing issues. Pair programming or code review sessions can uncover blind spots and foster collective problem-solving;
- Document Debugging Steps: Maintain documentation or notes detailing the steps taken during debugging sessions, including any hypotheses, findings, or resolutions reached. This documentation serves as a valuable reference for future troubleshooting efforts.
5. Tapping into Documentation and Community Support
When grappling with parsing challenges, tapping into available documentation and community resources can provide invaluable assistance. Here’s how to leverage these resources effectively:
- Thoroughly Review Library Documentation: Dive deep into the documentation provided by the JSON parsing library you’re utilizing. Look for troubleshooting guides, FAQs, and best practices sections that offer insights into common parsing pitfalls and solutions;
- Engage Online Forums and Communities: Join developer forums and communities like Stack Overflow, where you can seek guidance from experienced developers facing similar parsing issues. Utilize search functionalities to find existing threads discussing relevant topics or post specific queries for tailored assistance;
- Contribute and Share Knowledge: Actively participate in online communities by sharing your own experiences and insights related to JSON parsing. By contributing valuable information and solutions, you not only help others but also enhance your own understanding and problem-solving skills;
- Explore Sample Code and Tutorials: Seek out sample code snippets and tutorials related to JSON parsing to gain practical insights and learn from real-world implementations. Analyze and adapt these resources to suit your specific parsing requirements and challenges;
- Stay Open to New Techniques: Remain open-minded and adaptive in your approach to parsing problem-solving. Explore alternative techniques, libraries, or methodologies suggested by documentation or community members to broaden your troubleshooting toolkit.
Conclusion
Mastering JSON parsing in Java is indispensable for seamlessly engaging with APIs, delving into configuration files, and manipulating data. Although org.json presents a straightforward approach, alternate frameworks such as Jackson and Gson present a gamut of sophisticated functionalities coupled with superior performance. The choice of library hinges on individual exigencies and inclinations, necessitating a judicious selection that aligns seamlessly with the project’s requisites. Armed with insights garnered from this discourse, you embark on the journey of parsing JSON data in Java with a newfound assurance.