Within the realm of Java programming, the need to convert a double value to an integer arises in diverse situations. This necessity may manifest when one seeks to truncate the decimal component of a numerical value or when engaged in calculations reliant on integer data. Luckily, Java offers several streamlined and precise methods to accomplish this conversion task. Let us delve into the exploration of some prevalent methodologies for achieving this objective.
Leveraging Type Casting in Java
Type casting stands as a fundamental technique in Java programming, enabling the conversion of variables from one data type to another. Among its applications, one notable use is converting a double to an int. This process, while straightforward, requires precision to ensure accurate results. By implementing type casting, programmers can seamlessly manipulate data types, catering to various computational needs.
Process Overview: Type Casting
Type casting facilitates the conversion of a variable from a wider data type (such as double) to a narrower one (such as int). When transforming a double to an int, Java automatically truncates the decimal portion, effectively rounding down the number. The following steps outline the process:
- Define the Original Double Value: Begin by assigning a double value to a variable. This value represents the initial data to be converted;
- Perform Type Casting: Utilize type casting syntax, encapsulating the double value within parentheses followed by the target data type (int). This action instructs Java to perform the conversion;
- Retrieve Converted Value: Assign the result of the type casting operation to an integer variable, capturing the truncated double value;
- Display the Outcome: Output the converted integer value to the console, providing visibility into the transformation.
Implementation Example:
double doubleValue = 10.5;
int intValue = (int) doubleValue;
System.out.println("Converted int value: " + intValue); // Output: 10
Exploring Math Rounding Methods in Java
Java’s Math class offers a set of powerful tools for rounding double values to integers, catering to various needs and scenarios. Whether you need precise floor, ceiling, or rounded values, Java’s Math.floor(), Math.ceil(), and Math.round() methods have got you covered.
Math.floor():
Functionality: This method returns the largest double value that is less than or equal to the given argument and then converts it into an integer.
Usage Example:
double doubleValue = 10.5;
int intValue = (int) Math.floor(doubleValue);
System.out.println("Converted int value using Math.floor(): " + intValue); // Output: 10
Insights & Tips:
- Ideal for situations where you need to round down to the nearest integer;
- Particularly useful in scenarios like financial calculations, where precision is paramount;
- Note that Math.floor() truncates the fractional part towards negative infinity.
Math.ceil():
Functionality: This method returns the smallest double value that is greater than or equal to the given argument and then casts it to an integer.
Usage Example:
double doubleValue = 10.5;
int intValue = (int) Math.ceil(doubleValue);
System.out.println("Converted int value using Math.ceil(): " + intValue); // Output: 11
Insights & Tips:
- Perfect for rounding up to the nearest integer;
- Often employed in situations where you need to ensure a minimum value or allocate resources;
- Math.ceil() rounds the fractional part towards positive infinity.
Math.round():
Functionality: This method rounds the double value to the nearest integer. In cases where the fractional part is precisely halfway between two integers, it rounds towards the nearest even integer.
Usage Example:
double doubleValue = 10.5;
int intValue = (int) Math.round(doubleValue);
System.out.println("Converted int value using Math.round(): " + intValue); // Output: 11
Insights & Tips:
- Offers balanced rounding, making it suitable for various applications;
- Useful in scenarios where you require a more natural, intuitive rounding mechanism;
- Math.round() is often used in graphical user interfaces for pixel positioning and animation timing.
Considerations:
- Precision vs. Performance: Choose the rounding method that best aligns with your precision needs while considering computational efficiency;
- Handling Negative Values: Remember that rounding can behave differently for negative numbers, so ensure your implementation handles them appropriately;
- Documentation: Always refer to Java’s official documentation for detailed information and examples on Math rounding methods;
- Testing: Verify the behavior of rounding methods with comprehensive testing, covering edge cases and boundary conditions.
Utilizing Double.intValue() Method in Java
In Java programming, the Double class offers a versatile method known as intValue(), which serves the purpose of converting a double value into an integer, returning its integer representation. This conversion facilitates seamless manipulation and usage of numeric data, especially in scenarios where integers are required rather than doubles.
// Example Usage:
double doubleValue = 10.5;
int intValue = Double.valueOf(doubleValue).intValue();
System.out.println("Converted int value using Double.intValue(): " + intValue); // Output: 10
Expanding on the functionality and significance of Double.intValue(), let’s delve into its workings and explore its applications in Java development:
- Precision Handling: When dealing with floating-point numbers, precision issues may arise due to their inherent nature. By employing intValue(), developers can mitigate such concerns by converting double values to integers, ensuring precise calculations and results;
- Data Type Conversion: In scenarios where an integer representation of a double value is required, intValue() serves as a handy tool for seamlessly converting between data types, enhancing code readability and efficiency;
- Mathematical Operations: For arithmetic operations that necessitate integer operands, intValue() facilitates the transformation of double values into compatible integer types, enabling straightforward mathematical computations without the complexity of dealing with floating-point numbers;
- Compatibility with Integer-Based Functions: Many Java APIs and libraries expect integer inputs for certain functions or methods. By utilizing intValue(), developers can easily adapt double values to meet such requirements, ensuring compatibility and interoperability within their codebase;
- Error Handling: During numeric conversions, potential errors such as overflow or underflow may occur. It’s essential for developers to handle such scenarios gracefully to prevent runtime exceptions. Proper error handling techniques should be employed, such as try-catch blocks, to manage any exceptions that may arise during the conversion process;
- Performance Considerations: While intValue() provides convenience in converting double values to integers, developers should be mindful of its performance implications, especially when dealing with large datasets or frequent conversions. Profiling tools can be employed to identify and optimize any performance bottlenecks associated with its usage;
- Alternative Approaches: While intValue() is a straightforward method for converting double values to integers, developers may also explore alternative approaches depending on specific use cases. Techniques such as casting or rounding can offer different behaviors and precision levels, providing flexibility in numeric data manipulation.
Addressing Double to Int Conversion Issues in Java
1. Loss of Precision
Converting a double to an int in Java can lead to loss of precision due to the differing capabilities of representing values between the two data types. This loss is particularly notable when the double value contains a fractional part or exceeds the range of valid int values. Here’s an in-depth look at handling this issue:
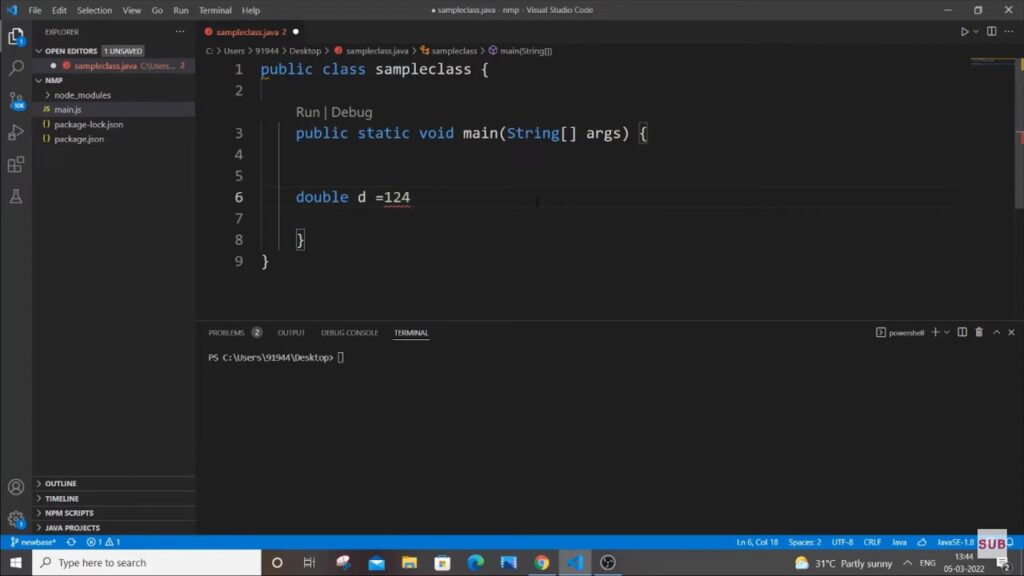
Understanding the Problem: The int data type in Java can only represent whole numbers within a specific range (-2^31 to 2^31-1), whereas doubles can accommodate a broader spectrum of values, including fractions and larger numbers.
Common Scenario: Consider the following example:
double doubleValue = 1234567890123456.789;
int intValue = (int) doubleValue;
System.out.println("Converted int value: " + intValue); // Output: -2147483648 (Integer overflow)
- Solution Approach:
- Range Validation: Ensure that the double value falls within the acceptable range of int values before conversion;
- Error Handling: Implement mechanisms to handle overflow scenarios gracefully, such as by checking the range before conversion or employing exception handling.
2. Managing NaN and Infinity
When dealing with double values in your Java code, encountering NaN (Not a Number) or Infinity can lead to unexpected outcomes during conversion to integer types. These special cases require careful handling to ensure your program behaves as expected.
Understanding NaN and Infinity
NaN and Infinity are special values in Java’s floating-point arithmetic:
- NaN (Not a Number): Represents the result of undefined or indeterminate operations, such as 0/0;
- Infinity: Represents values that exceed the range of representable floating-point numbers.
3. Handling NaN and Infinity
Before converting double values to integers, it’s crucial to check for NaN and Infinity using the appropriate methods provided by the Double class:
- Double.isNaN(): Checks if the value is NaN;
- Double.isInfinite(): Checks if the value is infinite.
Best Practices
To manage NaN and Infinity effectively:
- Check for special cases before conversion;
- Handle NaN and Infinity appropriately to avoid unexpected outcomes;
- Implement conditional logic to address these cases in your code flow.
double doubleValue = Double.NaN;
int intValue;
if (Double.isNaN(doubleValue) || Double.isInfinite(doubleValue)) {
// Handle special cases here
intValue = 0; // Example: Assign a default value
} else {
// Perform regular conversion
intValue = (int) doubleValue;
}
System.out.println("Converted int value: " + intValue);
By proactively addressing NaN and Infinity scenarios, you can ensure robustness and reliability in your Java applications.
4. Navigating Type Casting Challenges
Type casting in Java allows you to convert variables from one data type to another. However, it’s essential to be aware of potential pitfalls, especially regarding truncation behavior, which can differ from rounding methods like Math.round().
Understanding Type Casting
Type casting involves converting a value from one data type to another. When casting from a floating-point type to an integer type, truncation occurs, where the fractional part of the number is discarded.
Truncation Pitfalls: Consider the following example:
double doubleValue = -10.5;
int intValue = (int) doubleValue;
System.out.println("Converted int value: " + intValue); // Output: -10 (Truncates towards zero)
In this case, the decimal part (-0.5) is discarded, leading to -10 instead of -11.
Choosing the Right Approach
To avoid unexpected results when converting between data types:
- Be mindful of truncation: Understand that type casting truncates towards zero;
- Consider rounding methods: Depending on your requirements, alternatives like Math.round() may be more suitable for rounding floating-point values.
Best Practices
When dealing with type casting challenges:
- Assess the impact of truncation on your calculations;
- Use appropriate rounding methods when precise rounding is necessary;
- Verify the behavior of type casting in edge cases to ensure accuracy.
double doubleValue = -10.5;
int intValue = (int) doubleValue; // Truncates towards zero
int roundedValue = (int) Math.round(doubleValue); // Rounds to the nearest integer
System.out.println("Truncated int value: " + intValue);
System.out.println("Rounded int value: " + roundedValue);
By understanding the nuances of type casting and selecting the right approach, you can mitigate potential errors and ensure the integrity of your Java code.
Conclusion
Transforming a double into an integer in Java emerges as a frequent endeavor, offering multiple avenues to achieve this depending on the nuances of your requirements. Whether your inclination leans towards straightforward type casting or exact rounding facilitated by Math functions, Java grants the versatility necessary to navigate such conversions adeptly. Proficiency in these techniques empowers you to discern and adopt the optimal methodology tailored to your programming exigencies.