Java Swing provides developers with the flexibility to customize the appearance of GUI components, including JLabels. JLabels are essential for displaying text or images in a graphical user interface. However, setting the font size and color of a JLabel requires specific steps to achieve the desired visual presentation. In this comprehensive guide, we will delve into the process of setting the font size and color of JLabels in Java Swing applications.
What is JLabel in Java Swing?
A JLabel in Java Swing serves as a display area for text strings or images. It is a fundamental GUI component that allows developers to present information to users in a visually appealing manner. Unlike interactive components, such as buttons or text fields, a JLabel does not respond to input events and cannot receive keyboard focus.
Importance of Customizing JLabel Appearance
Customizing the appearance of JLabels is crucial for enhancing the overall aesthetics and readability of an application’s user interface. By adjusting the font size and color of JLabels, developers can create visually engaging displays that effectively convey information to users.
Example Source Code: Setting JLabel Font Size
JLabel jUserName = new JLabel("Demo: How to Set JLabel Font Size");
jUserName.setFont(new Font("Serif", Font.BOLD, 11));
JFrame frame = new JFrame("Demo Window");
frame.add(jUserName);
frame.setVisible(true);
Setting Font Size for JLabels
To change the font size of a JLabel, developers can utilize the setFont() method along with the Font class in Java. By specifying the desired font type, style, and size, it is possible to customize the text displayed by the JLabel.
Steps to Set Font Size:
- Create a new JLabel instance;
- Use the setFont() method to set the desired font properties;
- Add the JLabel to a container, such as a JFrame, to display it within the application.
Font Sizes for JLabels
Font Size | Description |
---|---|
10 | Small |
12 | Medium |
14 | Large |
16 | Extra Large |
Benefits of Adjusting Font Size
By adjusting the font size of JLabels, developers can improve the readability of text content within an application. Choosing an appropriate font size ensures that information is presented clearly and legibly to users, enhancing the overall user experience.
Example Code Snippet: Setting Font Size
jUserName.setFont(new Font(“Arial”, Font.PLAIN, 14));
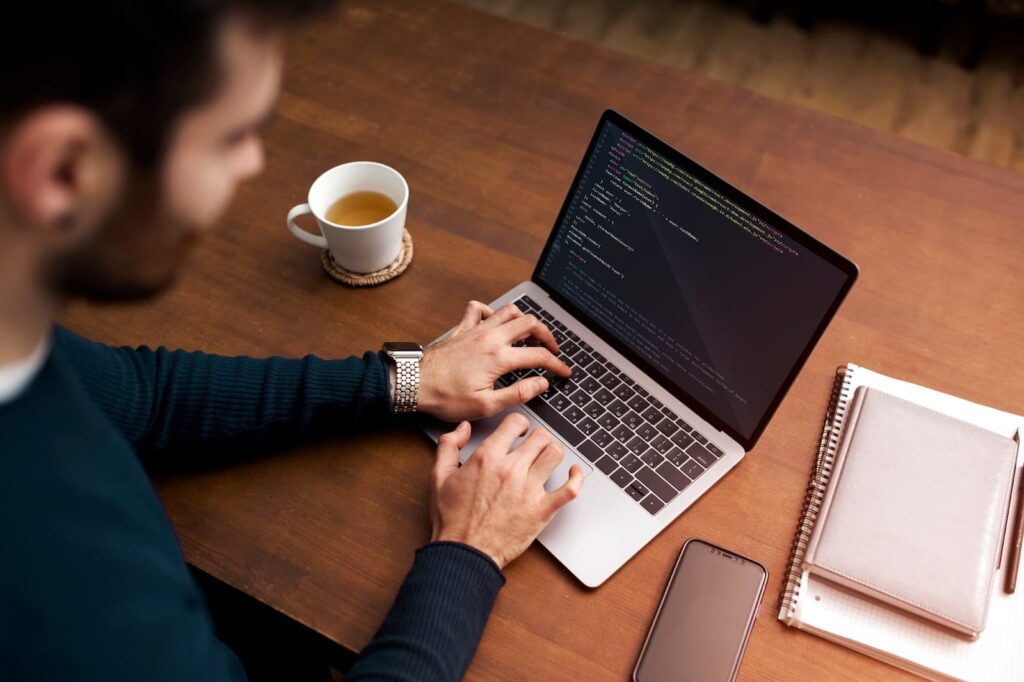
Customizing Font Color for JLabels
In addition to adjusting the font size, developers can also customize the text color of JLabels to create visually distinct elements within their applications. By changing the foreground color of a JLabel, it is possible to highlight important information or add visual emphasis to specific text content.
Steps to Set Font Color:
- Create a new JLabel instance;
- Utilize the setForeground() method to specify the desired text color;
- Add the JLabel to a container to display the text with the customized color.
Commonly Used Text Colors
- Red;
- Blue;
- Green;
- Black;
Advantages of Customizing Font Color
Customizing the font color of JLabels enables developers to create visually appealing interfaces that engage users and draw attention to key information. By strategically selecting text colors, developers can enhance the overall design aesthetics of their applications.
Code Example: Changing Font Color
jUserName.setForeground(Color.RED);
Enhancing JLabel Appearance with Background Color
While JLabel components have transparent backgrounds by default, developers can indirectly adjust the background color by adding them to containers with custom background colors. By placing a JLabel within a JPanel and setting the panel’s background color, developers can create visually cohesive layouts.
Steps to Indirectly Set Background Color:
- Create a new JLabel instance;
- Instantiate a JPanel and set its background color;
- Add the JLabel to the JPanel to display it against the specified background color.
Available Background Colors
Color Name | RGB Value |
---|---|
Blue | (0, 0, 255) |
Green | (0, 128, 0) |
Yellow | (255, 255, 0) |
Gray | (128, 128, 128) |
Benefits of Custom Background Colors
By incorporating background colors for JLabels, developers can create visually appealing contrasts that improve the overall readability and aesthetic appeal of their applications. Custom background colors help differentiate JLabels within the user interface, making them more visually engaging.
Sample Code Snippet: Adding Background Color
titlePanel.setBackground(Color.BLUE);
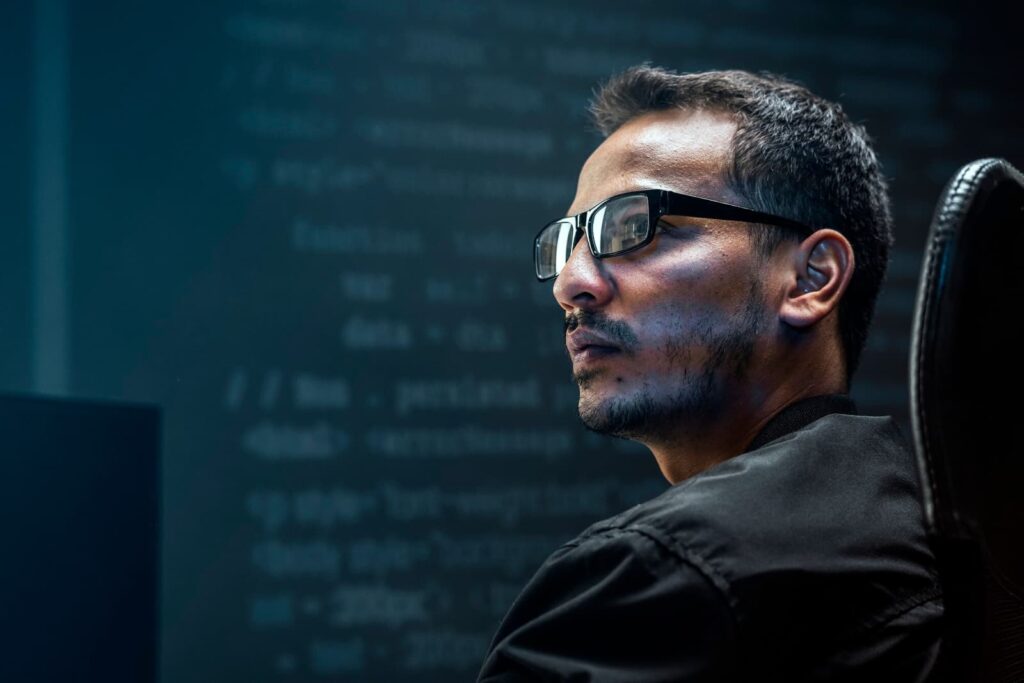
Advanced Techniques for JLabel Customization
Developers can explore external libraries and frameworks, such as JavaFX CSS or Apache Pivot, to implement advanced styling options for JLabels. These tools offer extensive customization capabilities, allowing developers to create sophisticated and visually appealing user interfaces.
Utilizing JavaFX CSS:
JavaFX CSS enables developers to apply CSS styles to JavaFX components, including JLabels, for enhanced visual effects and design flexibility. By leveraging CSS properties, developers can achieve intricate styling options beyond standard Java Swing features.
Animation Effects for Dynamic UI Elements
Integrating animation effects, such as transitions and fades, can further enhance the visual appeal of JLabels and create dynamic user interfaces. By incorporating subtle animations, developers can add interactivity and engagement to their applications, improving user experience.
Benefits of Animation Effects:
- Captivating user attention;
- Providing visual feedback for user interactions;
- Enhancing the overall aesthetic appeal of the application.
Conclusion
In conclusion, customizing the font size and color of JLabels in Java Swing applications plays a pivotal role in creating visually appealing and user-friendly interfaces. By following the outlined guidelines and best practices, developers can enhance the readability, aesthetics, and accessibility of their applications. Leveraging advanced techniques and external resources allows developers to push the boundaries of JLabel customization and create innovative user experiences. Mastering the JLabel customization empowers developers to craft compelling graphical interfaces that captivate users and elevate the overall quality of their applications.