JoptionPane is a class in the Swing library of Java that provides a set of pre-built dialog boxes for user input and output. These dialog boxes are commonly used in graphical user interface (GUI) applications to prompt the user for information or display messages. One of the most commonly used dialog boxes is the Showinputdialog, which allows the user to enter text into a text field. However, in some cases, we may need to ask for sensitive information such as passwords. In this article, we will explore how to use JoptionPane Showinputdialog with password and its implementation in Java.
Understanding JoptionPane Showinputdialog
Before diving into the implementation, let’s first understand how JoptionPane Showinputdialog works. The basic syntax for creating a Showinputdialog is as follows:
JOptionPane.showInputDialog(parentComponent, message);
Here, parentComponent refers to the parent component of the dialog box, and message is the message displayed to the user. This method returns a string value entered by the user. However, in our case, we need to ask for both a username and password. To achieve this, we can use the following syntax:
JOptionPane.showInputDialog(parentComponent, message, title, messageType);
Here, title refers to the title of the dialog box, and messageType specifies the type of message to be displayed. We can also customize the buttons displayed in the dialog box using the optionType parameter. Now, let’s see how we can add a password field to this dialog box.
Adding a Password Field to JoptionPane Showinputdialog
To add a password field to the Showinputdialog, we need to use the JPasswordField class, which is a subclass of JTextField specifically designed for password input. The basic syntax for creating a password field is as follows:
JPasswordField passwordField = new JPasswordField();
To add this password field to our Showinputdialog, we can use the add() method of the JOptionPane class. Let’s take a look at an example:
JLabel jUserName = new JLabel("User Name");
JTextField userName = new JTextField();
JLabel jPassword = new JLabel("Password");
JPasswordField password = new JPasswordField();
Object[] ob = ;
int result = JOptionPane.showConfirmDialog(null, ob, "Please enter your credentials", JOptionPane.OK_CANCEL_OPTION);
if (result == JOptionPane.OK_OPTION) {
String userNameValue = userName.getText();
String passwordValue = password.getText();
// Here is some validation code
}
In this example, we have created two labels, one for the username and one for the password, along with their corresponding text fields. Then, we have added these components to an object array and passed it to the showConfirmDialog() method. This method displays the dialog box with an OK and Cancel button and returns the user’s choice as an integer value. If the user clicks on the OK button, we can retrieve the values entered in the text fields using the getText() method.
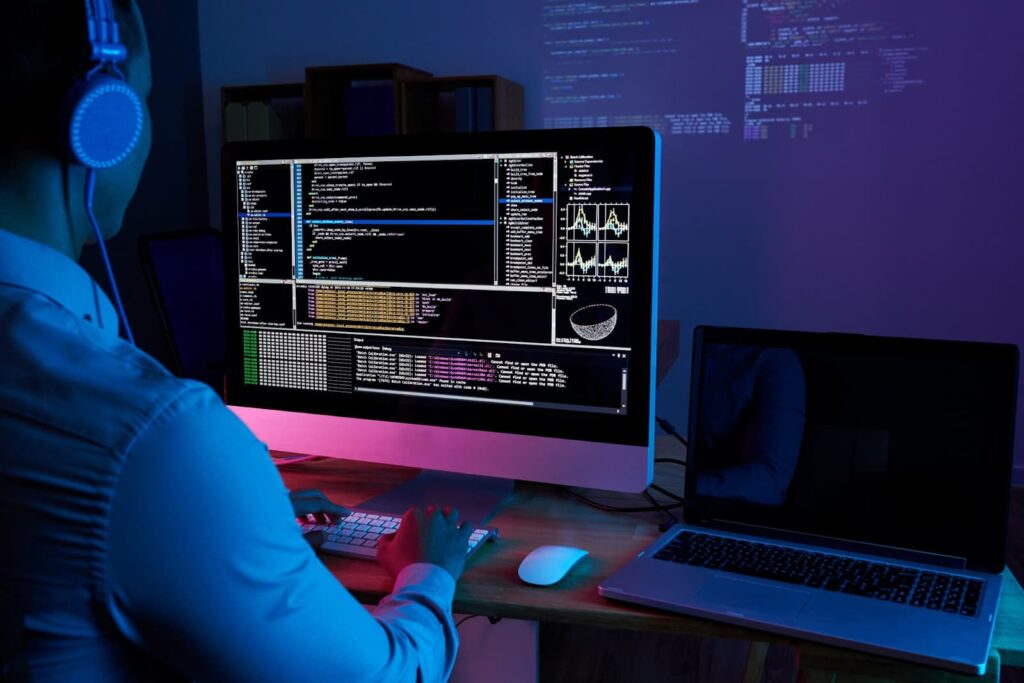
Implementing JoptionPane Showinputdialog With Password
Now that we have a basic understanding of how JoptionPane Showinputdialog works, let’s see how we can implement it in our Java code. We will create a simple login form that asks for a username and password and validates them against a predefined set of credentials.
Creating the GUI
First, we need to create a GUI for our login form. We will use the JFrame class to create a window and add the necessary components to it. Here’s the code for our GUI:
import javax.swing.*;
import java.awt.*;
public class LoginGUI extends JFrame {
private JLabel jUserName;
private JTextField userName;
private JLabel jPassword;
private JPasswordField password;
private JButton loginButton;
public LoginGUI() {
setTitle("Login Form");
setSize(400, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
// Create components
jUserName = new JLabel("User Name");
userName = new JTextField();
jPassword = new JLabel("Password");
password = new JPasswordField();
loginButton = new JButton("Login");
// Add components to the frame
setLayout(new GridLayout(3, 2));
add(jUserName);
add(userName);
add(jPassword);
add(password);
add(loginButton);
setVisible(true);
}
public static void main(String[] args) {
new LoginGUI();
}
}
In this code, we have created a JFrame object and added four components to it – two labels, two text fields, and a button. We have also set the layout of the frame to a grid layout with three rows and two columns. This will ensure that our components are displayed in an organized manner.
Adding Functionality to the Login Button
Next, we need to add functionality to our login button. When the user clicks on the button, we want to validate the username and password entered by the user. If they match our predefined credentials, we will display a success message; otherwise, we will display an error message. Here’s the code for our button’s action listener:
loginButton.addActionListener(e -> {
String userNameValue = userName.getText();
String passwordValue = password.getText();
if (userNameValue.equals("admin") && passwordValue.equals("password")) {
JOptionPane.showMessageDialog(null, "Login successful!");
} else {
JOptionPane.showMessageDialog(null, "Invalid credentials. Please try again.");
}
});
In this code, we have retrieved the values entered in the text fields and compared them with our predefined credentials. If they match, we display a success message using the showMessageDialog() method. Otherwise, we display an error message.
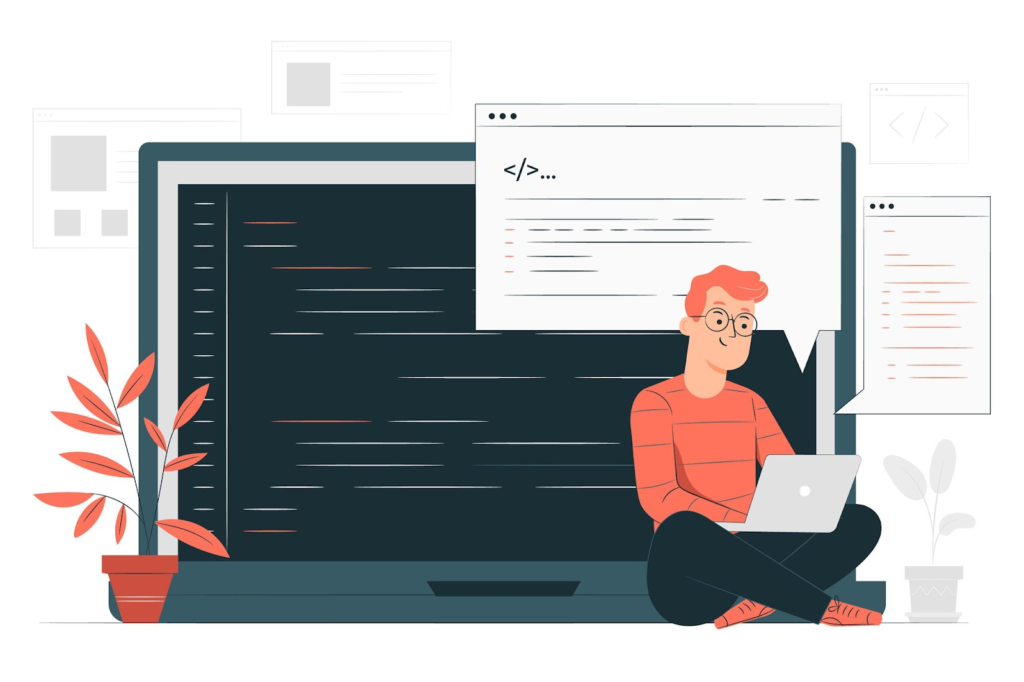
Creating a Register Dialog
Apart from a login form, we may also need to create a register form where users can sign up for our application. Let’s see how we can use JoptionPane Showinputdialog to create a register dialog.
Adding Components to the Dialog Box
First, we need to add the necessary components to our dialog box. We will use the same approach as before, but this time, we will add a password field instead of a text field. Here’s the code:
JLabel jFirstName = new JLabel("First Name");
JTextField firstName = new JTextField();
JLabel jLastName = new JLabel("Last Name");
JTextField lastName = new JTextField();
JLabel jEmail = new JLabel("Email");
JTextField email = new JTextField();
JLabel jPassword = new JLabel("Password");
JPasswordField password = new JPasswordField();
Object[] ob = ;
Validating User Input
Next, we need to validate the user input before registering them. We will check if all the fields are filled and if the email is in a valid format. We will also check if the password meets our criteria (at least 8 characters long and contains at least one uppercase letter, one lowercase letter, and one number). Here’s the code for our validation:
if (firstName.getText().isEmpty() || lastName.getText().isEmpty() || email.getText().isEmpty() || password.getPassword().length == 0) {
JOptionPane.showMessageDialog(null, "Please fill in all the fields.");
} else if (!email.getText().matches("[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]")) {
JOptionPane.showMessageDialog(null, "Please enter a valid email address.");
} else if (password.getPassword().length < 8 || !password.getText().matches(".*[A-Z].*") || !password.getText().matches(".*[a-z].*") || !password.getText().matches(".*[0-9].*")) {
JOptionPane.showMessageDialog(null, "Password must be at least 8 characters long and contain at least one uppercase letter, one lowercase letter, and one number.");
} else {
// Register user
}
In this code, we have used regular expressions to validate the email format and checked the password against our criteria. If any of the validations fail, we display an error message using the showMessageDialog() method.
Conclusion
In this article, we have explored how to use JoptionPane Showinputdialog with password in Java. We have seen how to add a password field to the dialog box and how to retrieve the values entered by the user. We have also implemented a simple login form and a register form using this dialog box. By now, you should have a good understanding of how JoptionPane Showinputdialog works and how you can use it in your own projects.