Welcome to the world of automated testing using JUnit 4! As a software developer, you know the importance of thorough testing in ensuring the quality and reliability of your code. However, writing test cases manually can be time-consuming and prone to human error. This is where JUnit comes in – a popular open-source framework that allows you to write and execute automated tests for Java code. In this tutorial, we will explore the basics of JUnit 4 and provide examples to help you get started with automated testing.
What is JUnit?
Automated testing is the process of running a program or script to automatically verify the behavior and functionality of an application. It involves creating test cases that simulate user actions and input, and comparing the actual output with the expected results. This helps developers catch bugs and errors early in the development cycle, reducing the cost and effort involved in fixing them at a later stage.
History and evolution of JUnit
JUnit was first introduced in 1997 by Kent Beck and Erich Gamma as a unit testing framework for the programming language Smalltalk. It was later rewritten and adapted for Java, becoming the de facto standard for automated testing in the Java community. JUnit 4 was released in 2006 and introduced several new features, making it more powerful and flexible than its predecessor.
Setting up JUnit
JUnit can be easily integrated into your Java project by adding the necessary dependencies to your build tool (e.g. Maven or Gradle). You can also download the JAR files from the official website and include them in your project manually. The latest version of JUnit 4 can be found at https://github.com/junit-team/junit4/releases.
IDE integration
Most popular Java IDEs like Eclipse, IntelliJ, and NetBeans have built-in support for JUnit. This makes it easy to create, run, and debug tests directly within your IDE. If you are using a different editor, you can still use JUnit by compiling your code with the JAR files included in your project.
Writing and executing tests
To create a test case in JUnit, you need to first annotate a method with the @Test annotation, which indicates that this method is a test case. For example:
@Test
public void testAddition() {
// Test code goes here
}
The @Test annotation can take optional parameters such as expected and timeout, which specify the expected result and maximum execution time for the test case, respectively.
Within the test method, you can use the various assertion methods provided by JUnit to verify the output of your code. Some commonly used assertions are assertEquals(), assertTrue(), and assertNotNull(). These methods take two parameters – the expected value and the actual value – and throw an AssertionError if they do not match.
For example:
@Test
public void testAddition() {
int result = Calculator.add(2, 3);
assertEquals(5, result);
}
Different types of assertions
JUnit provides a wide range of assertion methods to cater to different types of testing scenarios. Some common examples are:
- assertEquals(expected, actual): Checks if the expected and actual values are equal;
- assertNotEquals(unexpected, actual): Checks if the unexpected value is not equal to the actual value;
- assertTrue(boolean condition): Checks if the given condition evaluates to true;
- assertFalse(boolean condition): Checks if the given condition evaluates to false;
- assertNull(Object actual): Checks if the given object is null;
- assertNotNull(Object actual): Checks if the given object is not null.
You can find the complete list of assertion methods in the JUnit documentation.
Annotations and test fixtures
Annotations play a crucial role in JUnit as they provide metadata about your tests. We have already seen the @Testannotation, but JUnit also offers other useful annotations such as @Before, @After, and @Ignore.
The @Before and @After annotations are used to denote methods that need to be executed before and after each test case, respectively. This is useful when you want to perform setup and cleanup operations for every test case. For example:
@Before
public void setUp() {
// Code to initialize variables or objects
}
@Test
public void testAddition() {
// Test code goes here
}
@After
public void tearDown() {
// Code to clean up resources
}
The @Ignore annotation allows you to skip certain test cases without actually deleting them. This is helpful when a particular test case is failing, and you want to focus on fixing other issues first. Simply add @Ignore above the test method, and JUnit will skip it during execution.
Working with test fixtures
Test fixtures are used to set up a known state for your tests. This can be achieved using the @Before annotation, as shown in the previous section. However, JUnit also provides the @BeforeClass and @AfterClass annotations, which are used for setting up and cleaning up static objects that are shared across all test cases within a class. For example:
@BeforeClass
public static void setUpClass() {
// Code to initialize static objects or resources
}
@Test
public void testAddition() {
// Test code goes here
}
@AfterClass
public static void tearDownClass() {
// Code to clean up static objects or resources
}
Parameterized tests
JUnit allows you to write tests that take input parameters and run them multiple times with different inputs. This is useful when you have a method that behaves differently depending on the input values. To create a parameterized test, you need to use the @RunWith and @Parameterized annotations, along with a ParameterizedRunner class. Let’s see an example:
@RunWith(Parameterized.class)
public class CalculatorTest {
@Parameterized.Parameters
public static Collection data() {
return Arrays.asList(new Object[][]{
,
,
});
}
private int num1;
private int num2;
private int expectedResult;
public CalculatorTest(int num1, int num2, int expectedResult) {
this.num1 = num1;
this.num2 = num2;
this.expectedResult = expectedResult;
}
@Test
public void testAddition() {
int result = Calculator.add(num1, num2);
assertEquals(expectedResult, result);
}
}
In the above example, we have created a parameterized test for the Calculator class’s add() method. The @Parameterized.Parameters annotation specifies the input data in a 2D array, and each row represents different sets of parameters that will be passed to the test constructor.
Data-driven testing with JUnitParams
JUnitParams is an external library that provides more advanced features for creating parameterized tests. It allows you to use annotations like @Parameters, @CsvSource, and @ValueSource to specify the input data. Let’s take a look at an example:
@RunWith(JUnitParamsRunner.class)
public class CalculatorTest {
@Test
@Parameters()
public void testAddition(int num1, int num2) {
int result = Calculator.add(num1, num2);
assertTrue(result > 0);
}
@Test
@CsvSource()
public void testAdditionWithCsvInput(int num1, int num2, int expectedResult) {
int result = Calculator.add(num1, num2);
assertEquals(expectedResult, result);
}
@Test
@ValueSource(strings = )
public void testStringLength(String str) {
assertTrue(str.length() > 0);
}
}
The first test case uses the @Parameters annotation to specify the input data as comma-separated values. The second test case uses the @CsvSource annotation to provide input data in a more readable format. Lastly, the third test case uses the @ValueSource annotation to pass a single argument of different values to the test method.
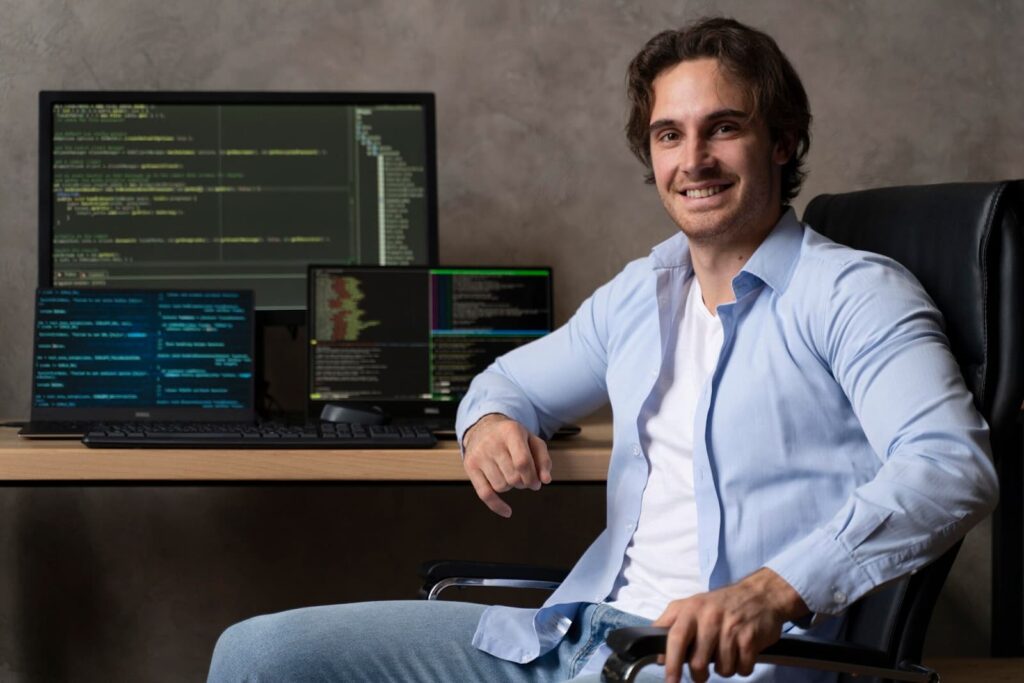
Advanced features
Test suites are used to group multiple test classes into a single test suite and run them all together. This is useful when you have a large project with several test classes, and you want to execute all tests at once. To create a test suite in JUnit, you need to use the @RunWith and @Suite annotations. Here’s an example:
@RunWith(Suite.class)
@Suite.SuiteClasses({
CalculatorTest.class,
StringUtilsTest.class
})
public class TestSuite {
}
JUnit also allows you to categorize your test cases using the @Category annotation. This is particularly useful when you want to exclude certain test cases from running during a build or specify different levels of tests (e.g. unit tests, integration tests). You can define your own categories and annotate your test methods or classes accordingly.
Exception handling and expected failures
JUnit provides a convenient way to test for exceptions by using the @Test(expected = Exception.class) annotation. This ensures that the specified exception is thrown during the execution of the test method. Additionally, you can also use the @Rule annotation to catch exceptions and handle them in a more sophisticated manner.
In some cases, you may want to deliberately fail a test to see if your code handles errors correctly. This can be achieved by using the fail() method provided by JUnit. For example:
@Test
public void testAdditionWithNegativeInputs() {
try {
int result = Calculator.add(-2, -3);
fail("Expected IllegalArgumentException not thrown");
} catch (IllegalArgumentException e) {
assertEquals("Inputs cannot be negative.", e.getMessage());
}
}
Conclusion
In this tutorial, we have covered the basics of JUnit 4 and provided examples to help you understand how to write and execute tests using this popular framework. We have explored different types of assertions, annotations, and advanced features such as parameterized tests and test suites. By now, you should have a good understanding of how JUnit works and how you can use it to automate your testing process.
JUnit is a versatile and powerful tool that can greatly improve the quality and reliability of your code. It is constantly evolving, with new features and updates being released frequently. So don’t be afraid to experiment and explore its capabilities further. Happy testing!